C Functions
GsCityDataGet
Retrieves data located with GsCityFindFirst() or GsCityFindNext().
Syntax
GsFunStat GsCityDataGet (GsId gs, intlu rec, GsEnum which,pstr pbuffer, int bufSize);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
intlurec City record number, as returned by GsCityFindFirst() or GsCityFindNext(). Input.
GsEnumwhich Symbolic constant for the data item to retrieve. Input.
The following table lists the valid GsEnums for most languages and the returned data sizes (including NULL characters).
GsEnum |
Size |
Description |
---|---|---|
GS_CITY_CARRTSORT |
(2) |
•Y – Carrier Route Sort •N – No Carrier Route Sort |
GS_CITY_CITYDELV |
(2) |
•Y – Post Office has city-delivery carrier routes •N – Post Office does not have city-delivery carrier routes |
GS_CITY_CLASS |
(2) |
ZIP Classification Code. |
GS_CITY_CTYSTKEY |
(7) |
Returns the 6-character USPS City State Key, which uniquely identifies a locale in the city/state file. |
GS_CITY_FACILITY |
(2) |
Returns the USPS City State Name Facility Code: •A – Airport Mail Facility (AMF) •B – Branch •C – Community Post Office (CPO) •D – Area Distribution Center (ADC) •E – Sectional Center Facility (SCF) •F – Delivery Distribution Center (DDC) •G – General Mail Facility (GMF) •K – Bulk Mail Center (BMC) •M – Money Order Unit •N – Non-Postal Community Name, Former Postal Facility, or Place Name •P – Post Office •S – Station •U – Urbanization |
GS_CITY_IS_ABBREV |
(2) |
•Y - If the current record is the 13-character USPS city name. •N - If the full city name. |
GS_CITY_MAILIND |
(2) |
•Y – Can use City State Name as last line on a mail piece •N – Cannot use City State Name as last line on a mail piece |
GS_CITY_NAME |
(30) |
City name. May be an alternative name. |
GS_CITY_PREFNAME |
(30) |
Returns the USPS preferred city name. |
GS_CITY_QCITY |
(10) |
City number; using the format ssffffccc, where s=state number, f=finance number, and c=city number. |
GS_CITY_UNIQUE |
(2) |
•Y – Unique ZIP Code (ZIP assigned to a single organization.) •Blank – Not applicable |
GS_CITY_STATE |
(3) |
State abbreviation |
GS_CITY_ZIP |
(6) |
ZIP Code |
pstrpbuffer Location to store the returned data. Output.
intbufSize Maximum size of data to return in the buffer. If bufSize is shorter than the data returned by GeoStan, GeoStan truncates the data and does not generate an error. Input.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsCityFindFirst() or GsCityFindNext()
Notes
The GsCityDataGet() function retrieves data about the city located with GsCityFindFirst() or GsCityFindNext(). GeoStan derives city information from the USPS City/State file.
Example
See GsCityFindFirst for an example.
GsCityFindFirst
Finds the first city matching the partial name or valid ZIP Code.
Syntax
intlu GsCityFindFirst(GsId gs, gs_const_str State,gs_const_str cityPattern);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
vgs_const_strState Various state abbreviations and spellings, or blank for a ZIP Code search. For example, for New Hampshire, it accepts:N HAMP, N HAMPSHIRE, NEW HAMPSHIRE, NEWHAMPSHIRE, NH, and NHAMPSHIRE. Input.
gs_const_strcityPattern City to search for (may be just a partial string), or a 3- or 5-digit ZIP Code. Input.
Return Values
Record number of the city located, or zero if GeoStan did not find any cities.
Prerequisites
GsClear()
Notes
This function retrieves the record number of the first city in a state that matches the city or ZIP Code search string. For example if the entered state string is CA and the city string is S, GeoStan finds the first city that begins with S in California. If the entered city string is 803 and the state string is null, GeoStan returns the first city in that sectional center. GeoStan does not return cities in any predefined order.
Before each find function, call GsClear() to reset the internal buffers. If you do not reset the buffers, you may receive incorrect results with information from a previous find.
Example
/* This example prints all city names in the 803 sectional center. */
intlu CityRecNum;
char CityName[30];
GsClear(gs);
CityRecNum = GsCityFindFirst( gs, "", "803" );
while (CityRecNum != 0 )
{
GsCityDataGet( gs, CityRecNum, GS_CITY_NAME, CityName,sizeof(CityName) );
printf( "%s\n", CityName );
GsClear(gs);
CityRecNum = GsCityFindNext( gs );
}
GsCityFindNext
Finds the next city matching the partial name or valid ZIP Code.
Syntax
intlu GsCityFindNext(GsId gs);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
Return Values
Record number of the located city, or zero if GeoStan did not find any cities.
Prerequisites
GsCityFindFirst() and GsClear()
Notes
Call this function after GsCityFindFirst().
Before each find function, call GsClear() to reset the internal buffers. If you do not reset the buffers, you may receive incorrect results with information from a previous find.
Example
See GsCityFindFirst.
GsClear
Clears the data buffer in the internal data structures.
Syntax
GsFunStat GsClear(GsId gs);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
Return Values
GS_SUCCESS
GS_ERROR
Notes
Before each find function, call GsClear() to reset the internal buffers to null. If you do not reset the buffers, you may receive incorrect results with information from a previous find. This call clears only address element and location information about the previously processed address and does not affect distance or centroid match type.
GsDataGet
Returns data for all address and matched elements from GeoStan.
Syntax
GsFunStat GsDataGet(GsId gs, GsEnum fInOut, GsEnum fSwitch,pstr pbuffer, intsu bufLen);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsEnumfInOut Flag indicating whether to retrieve original or processed data. Input.
To retrieve parsed address components, set fInOut to GS_INPUT. To retrieve matched address information from the parsed input address and the GeoStan database, set fInOut to GS_OUTPUT.
If the input address does not match, setting fInOut to GS_OUTPUT returns the address exactly as entered, except for the lastline information, which returns only the parsed lastline components.
The parsed lastline components correspond to the following enums:
GS_LASTLINE |
GS_ZIP4 |
GS_CITY |
GS_ZIP9 |
GS_STATE |
GS_ZIP10 |
GS_ZIP |
|
If there is extra data on the input lastline (GS_LASTLINE), this data is not retrievable. For example, in the lastline "BOULDER CO 80301 US OF A", "US OF A" is not retrievable from any GsDataGet() call.
To retrieve centerline return values, setting fInOut to GS_CENTERLINE returns a collection of ranged segments for streets that contain addresses. Individual addresses may be interpolated from the low-high ranges of the segments.
GsEnumfSwitch Symbolic constant for the data item to retrieve. Input.
pstrpbuffer Location to store the returned data. Output.
intsubufLen Maximum size of data for GeoStan to return. geostan.h lists as constants the recommended buffer sizes for each item. These sizes include the null terminator and are the maximum lengths required to get the full output string. You can allocate a buffer that is smaller or larger than these values. However, if bufLen is shorter than the returned data, GeoStan truncates the data and does not generate an error. Input.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsFindWithProps()
Notes
This function retrieves data elements from internal GeoStan buffers for either the original (input) or matched (output) data elements. To retrieve original data, set fInOut to GS_INPUT. To retrieve matched data, set fInOut to GS_OUTPUT.
If your GeoStan license has metering enabled, using GsDataGet() to return a geocode increments the counter unless the match code indicates an error or a 5-digit ZIP geocode.
GsDataSet
Passes data for all address elements to GeoStan.
Syntax
GsFunStat GsDataSet(GsId gs, GsEnum fSwitch, gs_const_str pBuffer);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsEnumfSwitch Symbolic constant for the data item to load. See Enums for storing and retrieving data for valid enums. Input.
gs_const_strpBuffer String pointer containing the data to load. Input.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsInitWithProps()
Notes
This function loads data elements into internal GeoStan buffers. When loading address information as a complete address or last line, GeoStan parses the data into fields. For example, if you enter a last line of "Boulder, CO 80301-1234", GeoStan parses the data and sets the city, state, ZIP, and ZIP + 4 fields. You can retrieve parsed input data using GsDataGet() with the GS_INPUT argument.
If you are passing both an address line and a last line or ZIP Code, enter the last line or ZIP Code first to ensure the greatest accuracy in address standardization.
If you are passing both the address information and the last line information as one input line, enter the address information first.
Using the appropriate GsEnums, you can pass singleline addresses, two-line addresses, or multiline addresses of up to six lines. For more information, see Appendix B: Extracting Data from GSD Files.
Do not call alter the GS_FIND_MATCH_MODE property value in your Find Properties after you have called GsDataSet(), because the previously established match mode affects how the input address is parsed. If you need to change the match mode in mid-process, you must re-enter the data for the current address with GsDataSet().
GsDpvGetCompleteStats
Syntax
GsFunStat GsDpvGetCompleteStats(GsId gs,GsDPVCompleteStats* pdata,int structSize);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsDPVCompleteStats*pdata Structure contains the following DPV statistics since the application initialized DPV.
intlu nCMRAInError |
Number of records with an unconfirmed CMRA. Output. |
intlu nCMRAValid |
Number of records with a confirmed CMRA. Output. |
intlu nDPVNoStatFound |
Not Applicable for this release. |
intlu nDPVInError |
Number of records not confirmed by DPV. Output. |
intlu nDPVSeedHits |
Number of distinct DPV false-positive matches. Output. |
intlu nDPVTypeDValid |
Number of records with a confirmed primary house number, but the secondary unit number is missing. Output. |
intlu nDPVTypeSValid |
Number of records with a confirmed primary house number, but the secondary unit number is not confirmed. Output. |
intlu nDPVTypeYValid |
Number of records fully confirmed by DPV. Output. |
intlu nDPVZipsOnFile |
Number of distinct ZIP Codes in file. Output. |
intlu nFirmCMRAPresented |
Number of USPS Firm records with PMB presented. Output. |
intlu nFirmCMRAValid |
Number of USPS Firm records CMRA confirmed with or without PMB. Output. |
intlu nFirmPrimeFail |
Number of USPS Firm records without a confirmed primary house number. Output. |
intlu nFirmSecondaryFail |
Number of USPS Firm records without a confirmed secondary unit number. Output. |
intlu nFirmValid |
Number of confirmed USPS Firm records. Output. |
intlu nFootnoteAA |
Number of records with DPV footnote value AA. Output. |
intlu nFootnoteA1 |
Number of records with DPV footnote value A1. Output. |
intlu nFootnoteBB |
Number of records with DPV footnote value BB. Output. |
intlu nFootnoteCC |
Number of records with DPV footnote value CC. Output. |
intlu nFootnoteF1 |
Number of records with DPV footnote value F1. Output. |
intlu nFootnoteG1 |
Number of records with DPV footnote value G1. Output. |
intlu nFootnoteM1 |
Number of records with DPV footnote value M1. Output. |
intlu nFootnoteM3 |
Number of records with DPV footnote value M3. Output. |
intlu nFootnoteN1 |
Number of records with DPV footnote value N1. Output. |
intlu nFootnoteP1 |
Number of records with DPV footnote value P1. Output. |
intlu nFootnoteP3 |
Number of records with DPV footnote value P3. Output. |
intlu nFootnoteRR |
Number of records with DPV footnote value RR. Output. |
intlu nFootnoteR1 |
Number of records with DPV footnote value R1. Output. |
intlu nFootnoteU1 |
Number of records with DPV footnote value U1. Output. |
intlu nGenDelValid |
Number of confirmed USPS General Delivery records. Output. |
intlu nHRCMRAPresented |
Number of USPS Highrise records with PMB presented. Output. |
intlu nHRCMRAValid |
Number of USPS Highrise records with confirmed CMRA records with or without PMB. Output. |
intlu nHRPrimeFail |
Number of USPS Highrise records without a confirmed primary house number. Output. |
intlu nHRSecondaryFail |
Number of USPS Highrise records without a confirmed secondary unit number. Output. |
intlu nHRValid |
Number of confirmed USPS Highrise records. Output. |
intlu nPOBoxFail |
Number of USPS PO Box records without a confirmed primary box number. Output. |
intlu nPOBoxValid |
Number of confirmed USPS PO Box records. Output. |
intlu nRRHCCMRAPresented |
Number of USPS Rural Route records with PMB presented. Output. |
intlu nRRHCCMRAValid |
Number of USPS Rural Route records CMRA confirmed with or without PMB. Output. |
intlu nRRHCPrimeFail |
Number of USPS Rural Route records without a confirmed primary house number. Output. |
intlu nRRHCValid |
Number of confirmed USPS Rural Route records. Output |
intlu nStCMRAPresented |
Number of USPS Street records with PMB presented. Output. |
intlu nStCMRAValid |
Number of USPS Street records with CMRA confirmed with or without PMB. Output. |
intlu nStreetPrimeFail |
Number of USPS Street records without a confirmed primary house number. Output. |
intlu nStreetSecondaryFail |
Number of USPS Street records without a confirmed secondary unit number. Output. |
intlu nStreetValid |
Number of confirmed DPV records that matched to a USPS street record (GS_REC_TYPE=S). Output. |
intlu nTotalDPVProcessed |
Number of records processed through DPV. Output. |
intlu nTotalDPVwithZip4 |
Number of ZIP + 4 records processed through DPV. Output. |
intstructSize Size of the GsDpvCompleteStats data structure. Input.
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx() for more information.
GS_WARNING Call GsErrorGetEx() for more information.
Prerequisites
GsInitDpv_r()
GsDpvGetFalsePosDetail
Retrieves the detail record for a DPV false-positive report.
Syntax
GsFunStat GsDpvGetFalsePosDetail(GsId gs,GsFalsePosDetailData* pdata,int structSize);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsFalsePosDetailData*pdata This structure retrieves the DPV detail record for the false-positive address match using the data passed in GsFalsePosDetailData. The data members are details provided by GeoStan for the false-positive report. This structure contains the following:
char AddressPrimaryNumber |
House number. Output. |
char AddressSecondaryAbbreviation |
Unit type (APT, SUITE, LOT). Output. |
char AddressSecondaryNumber |
Unit number. Output. |
char Filler |
Reserved for future implementation. Output. |
char MatchedPlus4 |
ZIP Code extension. Output. |
char MatchedZIPCODE |
ZIP Code. Output. |
char StreetName |
Name of the street. Output. |
char StreetPostDirectional |
Street name postdirectional (N, S, E, W). Output. |
char StreetpreDirectional |
Street name predirectional (N, S, E, W). Output. |
char StreetSuffixAbbreviation |
Street type (AVE, ST, RD). Output. |
intstructSize Size of theGsFalsePosDetailData data structure.Input.
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx() for more information.
GS_WARNING Call GsErrorGetEx() for more information.
Prerequisites
GsInitDpv_r()
Notes
Only valid if the current/last record is a DPV false-positive match: GS_DPV_FALSE_POS = "Y"
GsDpvGetFalsePosHeaderStats
Syntax
GsFunStat GsDpvGetFalsePosHeaderStats(GsId gs,GsFalsePosHeaderData *pdata, int structSize);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsFalsePosHeaderData *pdata This structure retrieves DPV statistics for the header record for false-positive address matches using the data passed in GsFalsePosHeaderData. The output values are statistics for the false-positive reports. The input values include information to correctly complete the false-positive report for the USPS. This structure contains the following:
char MailersAddressLine |
Address of the mailer. Input. |
char MailersCityName |
City of the mailer. Input. |
char MailerCompanyName |
Name of the mailer. Input. |
char MailersStateName |
State of the mailer. Input. |
char Mailers9DigitZip |
ZIP Code of the mailer. Input. |
intlu nNumberZipCodeOnFile |
Number of distinct ZIP Codes processed through DPV. Output. |
intlu nTotalRecordsMatched |
Number of records matched with DPV data. Output. |
intlu nTotalRecordsProcessed |
Number of records processed through DPV. Output. |
intlu nTotalRecordsZIP4Matched |
Number of records that have matched with ZIP + 4. Output. |
intlu NumberFalsePositives |
Number of found DPV false-positive matches. Output. |
intstructSize Size of theGsFalsePosHeaderDatadata structure. Input
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx() for more information.
GS_WARNING Call GsErrorGetEx() for more information.
Prerequisites
GsInitDpv_r()
GsErrorGet
Retrieves current error information.
Syntax
GsFunStat GsErrorGet(GsId gs, pstr pMessage, pstr pDetail);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
pstrpMessage Basic explanation for the error; up to 256 bytes in length. Output.
pstrpDetail Particulars of an error, such as filename; up to 256 bytes in length. Output.
Return Values
Error number of the most recent GeoStan error.
-1 |
No error. |
0 through 99 |
Indicates the actual DOS error values. |
100 |
Unclassified error. |
101 |
Unknown error. |
102 |
Invalid file signature. |
103 |
Table overflow. |
104 |
Insufficient memory. |
105 |
File not found. |
106 |
Invalid argument to a function. |
107 |
File is out of date. |
108 |
Invalid license filename, path, or incorrect password. |
109 |
Invalid GsFind call - cannot match an address and a geocode in the same GsFind call. |
110 |
Could not determine centerline. |
111 |
Invalid checksum on file contents. |
112 |
System exception (e.g. access violation). |
Alternates
GsErrorGetEx()
GsErrorGetEx
Retrieves GeoStan informational, error, and fatal warning information for the current thread.
Syntax
void GsErrorGetEx(GsID id, pstr error_message, pstr details)
Arguments
GsIDid GsId returned byGsInitWithProps() if initialization completed, or set to NULL if initialization failed and GsInitWithProps() returned NULL.
pstrerror_message GeoStan error code and descriptive text. The error_message has the following format: SGTppeeeDESCRIPTION, where:
nSGT is a constant value.
npp is the product number (01 for GeoStan).
neee is the three character error code.
nDESCRIPTION is a short text message naming the error.
pstrdetails Descriptive message, such as the file name associated with the error.
Return values
The next GeoStan error detected in the current thread. Upon return, error messages contain a brief description and additional text, such as the name of the file or directory associated with the error.
Prerequisites
GsInitWithProps()
GsErrorHas()
Example
See GsInit_r for example code.
GsErrorHas
Indicates if any errors occurred or any informational messages are available in the current thread.
Syntax
GsFunStat GsErrorHas(GsId id)
Arguments
GsIdid GsId returned byGsInitWithProps() if initialization completed, or set to NULL if initialization failed and GsInitWithProps() returned NULL. Input.
Return Values
True GeoStan generated errors or informational messages.
GSDFalse GeoStan did not generate any errors or informational messages
Prerequisites
GsInitWithProps()
Example
See GsErrorGetEx for example code.
GsFileStatus
Returns if the files successfully opened, and the date of the USPS data used in generating the primary GSD file.
Syntax
intsu GsFileStatus(GsId gs, gs_const_str stateCode, intsu *date);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
gs_const_strstateCode State FIPS or abbreviation (this argument is now ignored). Input.
intsu *date Publish date of the USPS data used in the current GeoStan release. Output.
Return Values
The return value is a series of bit-flags. The following GS_ENUMs are used to test these flags:
GS_FILE_EXISTS |
Opened a primary GSD file (us.gsd, use.gsd, usw.gsd). |
GS_CENTRUS_ALIAS_EXISTS |
Loaded the Centrus Alias file (usca.gsi). |
GS_SUPP_EXISTS |
Opened a supplemental GSD file (ust.gsd, uste.gsd, uswt.gsd). |
GS_STATEWIDE_EXISTS |
Initialized a state-wide intersection file* (us.gsi, use.gsi, usw.gsi).a |
GS_GSL_EXISTS |
Opened eLOT and Z4Change data b (us.gsl). |
GS_EWS_MATCH_EXISTS |
Loaded a valid, unexpired EWS file (ews.txt). |
GS_ZIPMOVE_EXISTS |
Opened ZIPMove data file (us.gsz). |
GS_ZIP9_IDX_EXISTS |
Opened ZIP9 index file (ZIP9.gsu, ZIP9e.gsu, ZIP9w.gsu). |
GS_AUXILIARY_EXISTS |
Opened an auxiliary file (.gax). |
GS_EXP_CENTROIDS_EXISTS |
Opened the Expanded Centroids file (us_cent.gsc - file included in the Master Location Structure Centroid Data Set (MLDB)). |
GS_REV_PBKEY_EXISTS |
Opened a Reverse PreciselyID Lookup file (*.pbk in DVDMLDR folder)a. |
a : You must have an additional license to use this file b: eLOT data requires an additional license. However, the Z4Change data is alwaysenabled. |
Prerequisites
GsInitWithProps()
Notes
The date argument indicates the creation date the primary GSD file. The date argument and the GS_INIT_RELDATE initialization property value can be evaluated as follows:
Year |
(date/384) + 1990 |
Month |
((date % 384) / 32) + 1 |
Day |
date % 32 |
Example
/* This example tests for the primary GSD file and eLOT files. */
intsu fileStatus, date;
fileStatus = GsFileStatus(gs, "", &date);
if(!(fileStatus & GS_GSL_EXISTS))
printf("eLOT file not initialized. \n");
if(!(fileStatus & GS_FILE_EXISTS))
printf("Primary GSD file not initialized.\n");
GsFileStatusEx
Returns information about the current GeoStan data set and license.
Syntax
intlu GsFileStatusEx(GsId gs, int mode, pstr buffer, int bufSize);
Arguments
GsIdgs ID returned by GsInitWithProps for the current instance of GeoStan. Input.
intmode Specific information to return. The following table includes the types of information available. Input.
See the Return Values section for information on the data types and datum returned.
Mode |
Data Returned |
---|---|
GS_STATUS_DATATYPE_NUM |
Returns a numeric constant representing the data type. |
GS_STATUS_DATATYPE_STR |
Returns GS_SUCCESS or GS_ERROR. GeoStan places this retrieved information in the buffer. |
GS_STATUS_DATUM_NUM |
Returns a numeric constant representing the datum. |
GS_STATUS_DATUM_STR |
This is the NAD used natively by the data. It does not reflect the datum currently in use by GeoStan. You can use the Find property, GS_FIND_CLIENT_CRS, to set the returned NAD. Returns GS_SUCCESS or GS_ERROR. The retrieved information is placed in the buffer. |
GS_STATUS_DAYS_REMAINING |
•For unmetered licenses and metered unlimited licenses, it returns DAYS_UNLIMITED or the number of days remaining before the expiration of the license. •For metered limited licenses, it returns the days remaining before license expiration. |
GS_STATUS_FILE_CHKSUM_NUM |
Returns a calculated value (an integer) used to check data integrity. Set the buffer and bufsize parameters to 0; they are unused. |
GS_STATUS_GEO_PRECISION |
Returns the number of decimal places of longitude/latitude stored in the GSD file. Default is 4. Point-level data is 6. |
GS_STATUS_GEO_RECORD_TOTAL |
•For unmetered licenses, it returns 0. •For metered licenses, it returns the total number of records geocoded. |
GS_STATUS_RECORDS_REMAINING |
•For unmetered licenses and metered licenses it returns RECORDS_UNLIMITED. •For metered limited licenses, it returns the number of records remaining on the license. Use GS_STATUS_DAYS_REMAINING, GS_STATUS_RECORDS_REMAINING, or GS_STATUS_GEO_RECORD_TOTAL to request information on the status of the GeoStan license. |
pstrbuffer Location to store the returned data. Output.
intbufSize Maximum size of data that GeoStan returns. If bufSize is shorter than the data returned by GeoStan, GeoStan truncates the data and does not generate an error. Input.
Return Values
This function returns a variety of information about the current GeoStan data set.
GS_STATUS_DATATYPE_X |
Lists the data vendor |
GS_STATUS_DATUM_X |
Returns the datum used to identify geographic coordinates. |
GS_STATUS_RECORDS_REMAINING, GS_STATUS_DAYS_REMAINING, GS_STATUS_GEO_RECORD_TOTAL |
Return license usage information. |
You can use the preceding arguments to determine the status of your GeoStan license, as shown in the GsInitWithProps() code example.
The following table shows the return values for GS_STATUS_DATATYPE_NUM.
GS_STATUS_DATATYPE_NUM |
Data Type |
---|---|
0 |
USPS |
1 |
TIGER |
2 |
TomTom street-level data |
4 |
Deprecated |
6 |
HERE (formerly NAVTEQ) street-level data |
7 |
TomTom point-level data |
9 |
Auxiliary file |
10 |
User Dictionary |
11 |
HERE (formerly NAVTEQ) point-level data |
12 |
Master Location Data |
The following table shows the return values for GS_STATUS_DATATYPE_STR.
GS_STATUS_DATATYPE_STR |
Data Type |
---|---|
USPS |
USPS |
TIGER |
TIGER |
TOMTOM |
TomTom street-level data |
HERE |
HERE street-level data |
TOMTOM POINT DATA |
TomTom point-level data |
AUXILIARY |
Auxiliary file |
USER DICTIONARY |
User Dictionary |
HERE POINT DATA |
HERE point-level data |
MASTER LOCATION |
Master Location Data |
The following table shows the return values for GS_STATUS_DATUM_STR.
GS_STATUS_DATUM_STR |
Data Type |
---|---|
0 |
NAD27 |
1 |
NAD83 (WGS84 for TomTom data) |
Prerequisites
GsInitWithProps()
GsFindFirst___
Finds the first street, segment, or range object that meets the search criteria.
Syntax
GsFunStat GsFindFirstRange(GsId gs, GsRangeHandle *pRange);
GsFunStat GsFindFirstSegment(GsId gs, GsSegmentHandle *pSegment);
GsFunStat GsFindFirstStreet(GsId gs, GsStreetHandle *pStreet,GsEnum option,gs_const_str pLocale,gs_const_str pName,gs_const_str pNumber);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsRangeHandle *pRange Pointer to a segment/range handle. Returns a valid handle if GeoStan finds a range. Input, Output.
GsSegmentHandle *pSegment Pointer to a street/segment handle. Returns a valid handle If GeoStan finds a segment. Input, Output.
GsStreetHandle *pStreet Pointer to a street handle. Returns a valid handle if GeoStan finds a street. Output.
GsEnumoption Determines the type of search performed by GeoStan. The following table lists the type of searches available. Input.
GS_ZIP_SEARCH |
GeoStan searches the ZIP Code specified by pLocale. When this option is set with GsFindFirstStreet(), GeoStan performs a finance search. |
GS_CITY_SEARCH |
GeoStan searches the city and state specified by pLocale. |
GS_EXTRA_SEARCH |
GeoStan searches the primary street files, Use.gsd and Usw.gsd, and the supplemental street files, Ustw.gsd and Uste.gsd, for streets without house numbers. If you specify this option, pNumber must be blank. If you specify pNumber, GeoStan searches only the primary street files, Usw.gsd and Use.gsd, which contain addressed segments. Assign the directory for this file to the GS_INIT_DATAPATH initialization property of the property list that you pass when calling GsInitWithProps(). MVS customers receive one primary street file, Us.gsd, and one supplemental street file, Ust.gsd. |
GS_SDX_SEARCH |
GeoStan searches by soundex. pName is a pointer to a numeric soundex key returned by GsSoundex. If you do not specify this option, GeoStan assumes that pName is a street name. |
You must specify either GS_ZIP_SEARCH or GS_CITY_SEARCH.
gs_const_strpLocale Sets the search area. If option is set to GS_ZIP_SEARCH, then pLocale is a valid ZIP Code. If option is set to GS_CITY_SEARCH, then pLocale is a valid city and state. GeoStan performs a city search by searching all finance areas that cover that city. This may result in found objects outside the actual city.Input.
gs_const_strpName Street name, or partial street name, for which to search. If option is set to GS_SDX_SEARCH, then pName is a pointer to a numeric soundex key. Input.
This limits the search to street names that begin with the name string. If pName is "APPLE," then GeoStan returns only streets beginning with Apple, such as Apple or Appleton. If pName is not specified, GeoStan finds all streets specified by pLocale.
gs_const_strpNumber House number that must be within a range. If option is set to GS_EXTRA_SEARCH, then pNumber must be blank. Input.
This returns only those ranges that contain that house number. This can be an alpha-numeric house number, such as 12N123W.
Return Values
GS_ERROR Error occurred. You can retrieve the error using GsErrorGet.
GS_LASTLINE_NOT_FOUND GeoStan could not find pLocale.
GS_NOT_FOUND GeoStan did not find a match.
GS_SUCCESS GeoStan found a match. You can retrieve the data using GsHandleGet().
Prerequisites
GsInitWithProps() and GsClear()
Notes
You must call GsFindFirstStreet() before GsFindFirstSegment() and GsFindFirstSegment() before GsFindFirstRange(). GsFindFirstStreet() also sets the area and criteria for subsequent segment and range searches.
If you are using GsFindFirstStreet() to find a street that has a number as a component of the street name, such as "US HWY 41" or "I-95," enter just the number, as the text is not part of the index for such streets.
GsFindFirst___ and GsFindNext___ functions work together to allow you to access to the entire address directory database.
See Appendix B: Extracting Data from GSD Files for more information on the Find First functions and segment and range searches.
Example
/* This example searches ZIP Code 80301 for streets beginning with "A" and no * specific house number. */
char tempstr[60];
GsStreetHandle hStreet;
GsSegmentHandle hSegment;
GsRangeHandle hRange;
/* Use GsFindFirstStreet to get the first street handle that matches the criteria. */
int iStat = GsFindFirstStreet(gs, &hStreet, GS_ZIP_SEARCH, "80301", "A", "");
while(iStat == GS_SUCCESS)
{
/* We have a valid street handle, which we convert to a range handle so that we can use GsHandleGet to retrieve information to show the user. Even though we converted to a range handle, it really is still just a street handle, so we can only access those elements that are valid at the street level. See Appendix A for a complete list of valid elements for each handle type.*/
hSegment.hStreet = hStreet;
hRange.hSegment = hSegment;
GsHandleGet(gs, GS_PREDIR, &hRange, tempstr,sizeof(tempstr));
printf("Street: %s ", tempstr);
GsHandleGet(gs, GS_NAME, &hRange, tempstr, sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet( gs, GS_TYPE, &hRange, tempstr, sizeof(tempstr) );
printf("%s ", tempstr);
GsHandleGet(gs, GS_POSTDIR, &hRange, tempstr,sizeof(tempstr));
printf( "%s\n", tempstr);
/* find the first valid segment for the current street*/
iStat = GsFindFirstSegment(gs, &hSegment);
while(iStat == GS_SUCCESS)
{
/* We have a valid segment, convert to a range handle to get information to display.*/
hRange.hSegment = hSegment;
printf(" Segment: " );
GsHandleGet(gs, GS_BLOCK_LEFT, &hRange, tempstr, sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet(gs, GS_BLOCK_RIGHT, &hRange, tempstr, sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet(gs, GS_SEGMENT_PARITY, &hRange, tempstr, sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet(gs, GS_SEGMENT_DIRECTION, &hRange, tempstr, sizeof(tempstr));
printf("%s \n", tempstr);
/* Get the first valid range for the current segment. */
iStat = GsFindFirstRange(gs, &hRange);
while(iStat == GS_SUCCESS)
{
GsHandleGet(gs, GS_LORANGE, &hRange, tempstr,sizeof(tempstr));
printf(" Range: %s ", tempstr);
GsHandleGet(gs, GS_HIRANGE, &hRange, tempstr,sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet(gs, GS_BLOCK_LEFT, &hRange, tempstr, sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet(gs, GS_PREDIR, &hRange, tempstr,sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet(gs, GS_NAME, &hRange, tempstr, sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet(gs, GS_TYPE, &hRange, tempstr, sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet(gs, GS_POSTDIR, &hRange, tempstr,sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet(gs, GS_ZIP, &hRange, tempstr, sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet(gs, GS_LOUNIT, &hRange, tempstr,sizeof(tempstr));
printf("%s ", tempstr);
GsHandleGet(gs, GS_HIUNIT, &hRange, tempstr,sizeof(tempstr));
printf("%s\n", tempstr);
/* Get the next valid range for this segment. */
iStat = GsFindNextRange(gs, &hRange);
}
if (iStat == GS_ERROR) break;
/* get the next valid segment for this street*/
iStat = GsFindNextSegment(gs, &hSegment);
}
if (iStat == GS_ERROR) break;
/* Get the next street that meets the initial criteria. */
iStat = GsFindNextStreet(gs, &hStreet);
}
GsFindFirstSegmentByMbr
Finds the first segment handle within the specified MBR.
Syntax
GsFunStat GsFindFirstSegmentByMbr(GsId gs, GsSegmentHandle *pSegment, long lon1,long lat1,long lon2,long lat2);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsSegmentHandle*pSegment Pointer to a street/segment handle. Returns a valid handle if GeoStan finds a segment. Input, Output.
longlon1 Minimum longitude. One of four values that make up the MBR. Input.
longlat1 Minimum latitude. One of four values that make up the MBR. Input.
longlon2 Maximum longitude. One of four values that make up the MBR. Input.
longlat2 Maximum latitude. One of four values that make up the MBR. Input.
Return Values
GS_ERROR Error occurred. You can retrieve the error using GsErrorGet().
GS_LASTLINE_NOT_FOUNDGeoStan could not find pSegment.
GS_NOT_FOUND GeoStan did not find a match.
GS_SUCCESS GeoStan found a match. If returned, pSegment is a valid segment handle that you can use with GsHandleGet(), GsHandleGetCoords(), or GsGetMbr().
Prerequisites
GsInitWithProps() and GsClear()
Notes
GeoStan handles all spatial query functions in ten-thousandths of degrees—not millionths of degrees as with GsDataGet().
Before each find function, call GsClear() to reset the internal buffers. If you do not reset the buffers, you may receive incorrect results with information from a previous find.
MBR Representation by Lat/Lon
GeoStan defines the minimum bounding rectangle by the four arguments given as lon1, lon2, lat1 and lat2, defined from the two pairs of intersecting points given as lat1/lon1 and lat2/lon2. A line between those points creates the diagonal of the rectangle as shown in the following figure.
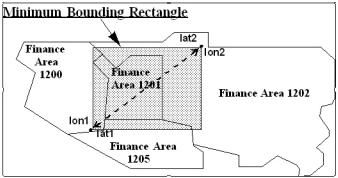
Example
#include <stdio.h>
#include <string.h>
#define GEOSTAN_PROPERTIES
#include "geostan.h"
const char *gsxOutPath = "c:\\temp";
/* This is a random MBR. */
const long minLon = -939460;
const long minLat = 438910;
const long maxLon = -936299;
const long maxLat = 440973;
/* This matches the spatialQueryProgress typedef in geostan.h. */
intl GS_STDCALL progress(void * param, intl mode, intl value)
{
static long totalSteps;
switch (mode)
{
case 0:
printf("%sStarting...\n", param);
totalSteps = value;
break;
case 1:
printf("%s%%%.2f complete\r", param, (double)value/
(double)totalSteps * 100);
break;
case 2:
printf("\n%sDone\n", param);
break;
}
/* In an interactive progress function, such as a dialog box, the user could choose to stop this operation. The correct return value would then be GS_PROGRESS_CANCEL. */
return GS_PROGRESS_CONTINUE;
}
int main(int argc, char **argv)
{
GsId gs;
PropList initProps;
PropList statusProps;
GsFunStat funStat;
GsSegmentHandle hSegment;
/* Initialization */
/* create an initialization property list with geostan information */
GsPropListCreate(&initProps, GS_INIT_PROP_LIST_TYPE);
/* GeoStan version */
GsPropSetLong(&initProps, GS_INIT_GSVERSION, GS_GEOSTAN_VERSION);
/* license filename and path */
GsPropSetStr(&initProps, GS_INIT_LICFILENAME, "c:\\lic\\geostan.lic");
/* license file password */
GsPropSetLong(&initProps, GS_INIT_PASSWORD, 43218765);
/* path to the data files, geostan library, and GSX files*/
GsPropSetStr(&initProps, GS_INIT_DATAPATH, "c:\\geostan\\datasets;c:\\geostan;c:\\temp");
/* path and file name of the ZIP Code geocoding data file*/
GsPropSetStr(&initProps, GS_INIT_Z4FILE, "c:\\geostan\\datasets\\us.z9");
/* GeoStan options */
GsPropSetBool(&initProps, GS_INIT_OPTIONS_ADDR_CODE, TRUE);
GsPropSetBool(&initProps, GS_INIT_OPTIONS_SPATIAL_QUERY, TRUE);
GsPropSetLong(&initProps, GS_INIT_CACHESIZE, 2);
/* create a status property list */
GsPropListCreate(&statusProps, GS_STATUS_PROP_LIST_TYPE);
gs = GsInitWithProps(&initProps, &statusProps);
if (!gs)
{
printf("Failed to initialize GeoStan library.\n");
return 1;
}
funStat = GsPrepareIndexMbr(gs, minLon, minLat, maxLon, maxLat, gsxOutPath, "MBR index preparation: ", progress);
if ( funStat == GS_ERROR )
{
printf("GsPrepareIndexMbr returned GS_ERROR.\n");
return 1;
}
funStat = GsFindFirstSegmentByMbr(gs, &hSegment, minLon, minLat, maxLon, maxLat);
while (funStat == GS_SUCCESS )
{
/* Insert code here to operate on each segment handle retrieved. */
funStat = GsFindNextSegmentByMbr(gs, &hSegment);
}
if (funStat == GS_ERROR)
{
printf("GsFindFirstSegmentByMbr or GsFindNextSegmentByMbr returned GS_ERROR.\n");
return 1;
}
GsTerm(gs);
GsPropListDestroy(&initProps);
GsPropListDestroy(&statusProps);
return 0;
GsFindFirstState
Finds the first state matching the name, abbreviation, or valid ZIP Code.
Syntax
GsFunStat GsFindFirstState (GsId gs, gs_const_str statePattern,pstr stateFound, int bufSize);
Arguments
GsId gs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
gs_const_strstatePattern Search string for the state. Input.
Can be any of the following items:
n A five-digit ZIP Code.
n The first three digits of a ZIP Code known as the Sectional Center Facility (SCF).
n Various state abbreviations and spellings. For example, for New Hampshire, it accepts: N HAMP, N HAMPSHIRE, NEW HAMPSHIRE, NEWHAMPSHIRE, NH, and NHAMPSHIRE.
pstrstateFound Proper state abbreviation for the located state. Output.
intbufSize Maximum length of data returned in stateFound, including NULL. Input.
Return Values
GS_ERROR
GS_SUCCESS
GS_NOT_FOUND
Prerequisites
GsInitWithProps() and GsClear()
Notes
This function returns the proper abbreviation for the requested state. You can request states by ZIP Code, full state name, or an alternate abbreviation
Before each find function, call GsClear() to reset the internal buffers. If you do not reset the buffers, you may receive incorrect results with information from a previous find.
Example
/* This example prints the official state abbreviation for ZIP Code 80301. */
char StateAbbr[3];
GsFunStat iStat;
iStat = GsFindFirstState(gs, "80301", StateAbbr, sizeof(StateAbbr));
if (iStat == GS_SUCCESS)
printf("%s\n", StateAbbr);
GsFindGeographicFirst
Finds the first city, county, and or state centroid match from the set of possible matches found.
Syntax
GsFunStat GsFindGeographicFirst(GsId gs, GsGeoStruct *gstruct);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsGeoStruct*gstruct Pointer to the GsGeoStruct containing the input and output fields for this API. Input, Output.
inCity City for which to search. Input.
inCounty County for which to search. Input.
inState State for which to search. Input.
outCity Output city. Output.
outCountry Output county. Output.
outState Output state. Output.
outLat Returned latitude of the geographic centroid. Output.
outLong Returned longitude of the geographic centroid. Output.
outRank Returned geographic rank of the city for city centroid. Output.
outResultCode Result code equivalent (G3 - city centroid, G2 - country centroid, G1 - state centroid). Output.
outLocCode Location code equivalent (GM - city, GC - county, GS - state). Output.
outClose True indicates a close match. Output.
Return Values
GS_SUCCESS
GS_ERROR
GS_NOT_FOUND
Prerequisites
GsInitWithProps()
Notes
It is recommended that the user first use the Last-line lookup functions to standardize the city, county and state names. This function only performs minimal fuzzy matching on the input city and county names. The location code returned by this function is to provide users with a location code equivalent and is not retrievable using GsDataGet. It is merely provided to offer a consistent label for the type of address match that is returned and will only consist of one of the three Geographic location codes (GM – City, GC – County and GS – State).
Example
To use this API, you will use the following structure defined in geostan.h.
/* The Geographic query structure. */
typedef struct GsGeoStruct {
char inCity[GS_CITY_CCS_LENGTH]; /* input city */
char inCounty[GS_COUNTY_CCS_LENGTH]; /* input county */
char inState[GS_STATE_CCS_LENGTH]; /* input state */
char outCity[GS_CITY_CCS_LENGTH]; /* output city */
char outCounty[GS_COUNTY_CCS_LENGTH]; /* output county */
char outState[GS_STATE_CCS_LENGTH]; /* output state */
char outLat[GS_LAT_LENGTH]; /* output latitude */
char outLong[GS_LON_LENGTH]; /* output longitude */
char outRank[GS_RANK_LENGTH]; /* output geographic rank */
char outResultCode[GS_MM_RESULT_CODE_LENGTH ]; /* output result code */
char outLocCode[GS_LOC_CODE_LENGTH]; /* output location code */
char outClose; /* output close match flag */
}GsGeoStruct;
An example of using the Geographic Geocoding API is as follows. This assumes you have a valid GsId named gs from a prior Geostan Initialization.
void GeographicGeocoding(GsId gs)
{
GsGeoStruct p;
int ret;
printf("Enter City : ");
gets(p.inCity);
printf("Enter County : ");
gets(p.inCounty);
printf("Enter State : ");
gets(p.inState);
ret = GsFindGeographicFirst(gs,&p);
if(ret != 0)
printf("No record found");
else
{
if(strcmp(p.outResultCode,"G3")==0)
printf("%s \t %s \t %s \t %s \t %s \n",
p.outCity,p.outState,p.outResultCode,p.outLat,p.outLong); if(strcmp(p.outResultCode,"G2")==0)
printf("%s \t %s \t %s \t %s \t %s \n",
p.outCounty,p.outState,p.outResultCode,p.outLat,p.outLong);
if(strcmp(p.outResultCode,"G1")==0)
printf("%s \t %s \t %s \t %s \n",
p.outState,p.outResultCode,p.outLat,p.outLong);
printf("\n");
while(ret==0)
{
ret=GsFindGeographicNext(gs, &p );
if(ret == 0)
{
if(strcmp(p.outResultCode,"G3")==0)
printf("%s \t %s \t %s \t %s \t %s \n",
p.outCity,p.outState,p.outResultCode,p.outLat,p.outLong);
if(strcmp(p.outResultCode,"G2")==0)
printf("%s \t %s \t %s \t %s \t %s \n",
p.outCounty,p.outState,p.outResultCode,p.outLat,p.outLong);
if(strcmp(p.outResultCode,"G1")==0)
printf("%s \t %s \t %s \t %s \n",
p.outState,p.outResultCode,p.outLat,p.outLong);
printf("\n");
}
}
}
}
GsFindGeographicFirstEx
Finds the first city, county, and or state centroid match from the set of possible matches found.
Syntax
GsFunStat GsFindGeographicFirstEx(GsId gs,GsGeoStructEx* pGeoStruct);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsGeoStructEx*pGeoStruct Pointer to the GsGeoStruct containing the input and output fields for this API. Input, Output.
inCity City for which to search. Input.
inCounty County for which to search. Input.
inState State for which to search. Input.
outCity Output city. Output.
outCountry Output county. Output.
outState Output state. Output.
outLat Returned latitude of the geographic centroid. Output.
outLong Returned longitude of the geographic centroid. Output.
outRank Returned geographic rank of the city for city centroid. Output.
outResultCode Result code equivalent (G3 - city centroid, G2 - country centroid, G1 - state centroid). Output.
outLocCode Location code equivalent (GM - city, GC - county, GS - state). Output.
inGeoLibVer GeoStan version. Input.
outClose True indicates a close match. Output.
outFipsCode FIPS Code. Output.
Return Values
GS_SUCCESS
GS_ERROR
GS_NOT_FOUND
Prerequisites
GsInitWithProps()
Notes
It is recommended that the user first use the Last-line lookup functions to standardize the city, county and state names. This function only performs minimal fuzzy matching on the input city and county names. The location code returned by this function is to provide users with a location code equivalent and is not retrievable using GsDataGet. It is merely provided to offer a consistent label for the type of address match that is returned and will only consist of one of the three Geographic location codes (GM – City, GC – County and GS – State).
Example
To use this API, you will use the following structure defined in geostan.h.
/* The Geographic query structure. */
typedef struct GsGeoStructEx{
char inCity[GS_CITY_CCS_LENGTH]; /* input city */
char inCounty[GS_COUNTY_CCS_LENGTH]; /* input county */
char inState[GS_STATE_CCS_LENGTH]; /* input state */
char outCity[GS_CITY_CCS_LENGTH]; /* output city */
char outCounty[GS_COUNTY_CCS_LENGTH]; /* output county */
char outState[GS_STATE_CCS_LENGTH]; /* output state */
char outLat[GS_LAT_LENGTH]; /* output latitude */
char outLong[GS_LON_LENGTH]; /* output longitude */
char outRank[GS_RANK_LENGTH]; /* output geographic rank */
char outResultCode[GS_MM_RESULT_CODE_LENGTH ]; /* output result code */
char outLocCode[GS_LOC_CODE_LENGTH]; /* output location code */
char outClose; /* output close match flag */
long inGeoLibVer; /* geostan version */
char outFipsCode[GS_FIPS_CCS_LENGTH]; /* output fips code */
} GsGeoStructEx;
An example of using the Geographic Geocoding API is as follows. This assumes you have a valid GsId named gs from a prior Geostan Initialization.
void GeographicGeocoding(GsId gs)
{
GsGeoStruct p;
int ret;
printf("Enter City : ");
gets(p.inCity);
printf("Enter County : ");
gets(p.inCounty);
printf("Enter State : ");
gets(p.inState);
ret = GsFindGeographicFirst(gs,&p);
if(ret != 0)
printf("No record found");
else
{
if(strcmp(p.outResultCode,"G3")==0)
printf("%s \t %s \t %s \t %s \t %s \n",
p.outCity,p.outState,p.outResultCode,p.outLat,p.outLong); if(strcmp(p.outResultCode,"G2")==0)
printf("%s \t %s \t %s \t %s \t %s \n",
p.outCounty,p.outState,p.outResultCode,p.outLat,p.outLong);
if(strcmp(p.outResultCode,"G1")==0)
printf("%s \t %s \t %s \t %s \n",
p.outState,p.outResultCode,p.outLat,p.outLong);
printf("\n");
while(ret==0)
{
ret=GsFindGeographicNext(gs, &p );
if(ret == 0)
{
if(strcmp(p.outResultCode,"G3")==0)
printf("%s \t %s \t %s \t %s \t %s \n",
p.outCity,p.outState,p.outResultCode,p.outLat,p.outLong);
if(strcmp(p.outResultCode,"G2")==0)
printf("%s \t %s \t %s \t %s \t %s \n",
p.outCounty,p.outState,p.outResultCode,p.outLat,p.outLong);
if(strcmp(p.outResultCode,"G1")==0)
printf("%s \t %s \t %s \t %s \n",
p.outState,p.outResultCode,p.outLat,p.outLong);
printf("\n");
}
}
}
}
GsFindGeographicNext
Finds the next city, county, and or state centroid match from the set of possible matches found.
Syntax
GsFunStat GsFindGeographicNext(GsId gs, GsGeoStruct *gstruct);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsGeoStruct*gstruct Pointer to the GsGeoStruct containing the input and output fields for this API. Input, Output.
outCity Output city. Output.
outCountry Output county. Output.
outState Output state. Output.
outLat Returned latitude of the geographic centroid. Output.
outLong Returned longitude of the geographic centroid. Output.
outRank Returned geographic rank of the city for city centroid. Output.
outResultCode Result code equivalent (G3 - city centroid, G2 - country centroid, G1 - state centroid). Output.
outLocCode Location code equivalent (GM - city, GC - county, GS - state). Output.
outClose True indicates a close match. Output.
Return Values
GS_SUCCESS
GS_ERROR
GS_NOT_FOUND
Prerequisites
GsInitWithProps()
Notes
It is recommended that the user first use the Last-line lookup functions to standardize the city, county and state names. This function only performs minimal fuzzy matching on the input city and county names. The location code returned by this function is to provide users with a location code equivalent and is not retrievable using GsDataGet. It is merely provided to offer a consistent label for the type of address match that is returned and will only consist of one of the three Geographic location codes (GM – City, GC – County and GS – State).
Example
See the example for GsFindGeographicFirst.
GsFindGeographicNextEx
Finds the next city, county, and or state centroid match from the set of possible matches found.
Syntax
GsFunStat GsFindGeographicNextEx(GsId gs, GsGeoStructEx* pGeoStruct);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsGeoStructEx*pGeoStruct Pointer to the GsGeoStruct containing the input and output fields for this API. Input, Output.
inCity City for which to search. Input.
inCounty County for which to search. Input.
inState State for which to search. Input.
outCity Output city. Output.
outCountry Output county. Output.
outState Output state. Output.
outLat Returned latitude of the geographic centroid. Output.
outLong Returned longitude of the geographic centroid. Output.
outRank Returned geographic rank of the city for city centroid. Output.
outResultCode Result code equivalent (G3 - city centroid, G2 - country centroid, G1 - state centroid). Output.
outLocCode Location code equivalent (GM - city, GC - county, GS - state). Output.
inGeoLibVer GeoStan version. Input.
outClose True indicates a close match. Output.
outFipsCode FIPS Code. Output.
Return Values
GS_SUCCESS
GS_ERROR
GS_NOT_FOUND
Prerequisites
GsInitWithProps()
Notes
It is recommended that the user first use the Last-line lookup functions to standardize the city, county and state names. This function only performs minimal fuzzy matching on the input city and county names. The location code returned by this function is to provide users with a location code equivalent and is not retrievable using GsDataGet. It is merely provided to offer a consistent label for the type of address match that is returned and will only consist of one of the three Geographic location codes (GM – City, GC – County and GS – State).
Example
See the example for GsFindGeographicFirstEx.
GsFindNext___
Finds the next street, segment, or range object that meets the search criteria.
Syntax
GsFunStat GsFindNextRange(GsId gs, GsRangeHandle *pRange);
GsFunStat GsFindNextSegment(GsId gs, GsSegmentHandle *pSegment);
GsFunStat GsFindNextStreet(GsId gs, GsStreetHandle *pStreet);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsRangeHandle*pRange Pointer to a segment/range handle. Returns a valid handle to the next range object, if there is one. Input, Output.
GsSegmentHandle*pSegment Pointer to a street/segment handle. Returns a valid handle to the next segment object, if there is one. Input, Output.
GsStreetHandle*pStreet Pointer to a street handle. Returns a valid handle to the next street object, if there is one. Input, Output.
Return Values
GS_SUCCESS
GS_NOT_FOUND
GS_ERROR
Prerequisites
GsFindFirst_ and GsClear()
Notes
This function continues to find objects matching the criteria specified in GsFindFirst__.
If GeoStan finds a matching segment, you can retrieve the data using GsHandleGet().
Before each find function, call GsClear() to reset the internal buffers. If you do not reset the buffers, you may receive incorrect results with information from a previous find.
Refer to Appendix B: Extracting Data from GSD Files for details on using all of the GsFindFirst___ and GsFindNext___ functions.
GsFindNextStreet() does not respect parity.
Example
See the code example in Appendix B: Extracting Data from GSD Files.
GsFindNextSegmentByMbr
Finds the next segment handle within the specified MBR.
Syntax
GsFunStat GsFindNextSegmentByMbr(GsId gs, GsSegmentHandle *pSegment);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsSegmentHandle*pSegment Pointer to a segment handle. Returns a valid handle to the next segment object, if there is one. Output.
Return Values
GS_ERROR Error occurred. You can retrieve the error using GsErrorGet.
GS_LASTLINE_NOT_FOUND GeoStan could not find pLocale.
GS_NOT_FOUND GeoStan did not find a match.
GS_SUCCESS GeoStan found a match. If returned, pSegment is a valid segment handle that you can use with GsHandleGet(), GsHandleGetCoords(), or GsGetMbr().
Prerequisites
GsFindFirstSegmentByMBR() and GsClear()
Notes
GeoStan handles all geocodes in the spatial query functions in ten-thousandths of degrees—not millionths like GsDataGet().
Before each find function, call GsClear() to reset the internal buffers. If you do not reset the buffers, you may receive incorrect results with information from a previous find.
Example
See GsFindFirstSegmentByMbr for a code example.
MBR Representation by Lat/Lon
See GsFindFirstSegmentByMbr for an MBR representation by latitude and longitude.
GsFindWithProps
Finds a match with user settable match preferences (properties).
Syntax
GsFunStat GsFindWithProps (GsId gs, PropList* pProps);
Arguments
GsId gs GeoStan handle. Input.
PropList*pProps Pointer to property list structure. Input.
Return Values
GS_ADDRESS_NOT_RESOLVED GeoStan cannot resolve a match candidate.
GS_ADDRESS_NOT_FOUND GeoStan did not find an address match or if you have a metered license and the GeoStan record count is depleted.
For metered licenses, GsFind decrements the GeoStan record counter in the license each time it returns a non-error match code. If the counter reaches zero or below, GsFind returns GS_ADDRESS_NOT_FOUND and sets the match code to E015. If you have an unmetered license, no decrementing occurs, and processing is unlimited.
GS_ERROR Low-level errors; use GsErrorGet to retrieve the error information.
GS_LASTLINE_NOT_FOUND GeoStan did not find a match for city/state or ZIP Code.
GS_NOT_FOUND GeoStan did not find a match for an input PreciselyID with Reverse PreciselyID Lookup.
GS_NOT_LICENSED Missing an optional feature license.
GS_SUCCESS GeoStan found a match.
Prerequisites
GsPropListCreate()
GsPropSet*()
Notes
The application owns the property list, but GeoStan is the active user.
Do not destroy the property list while GeoStan is still using that property list.
See table GsFindWithProps properties.
Example
GsFunStat retval;
PropList findProps;
GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
GsPropSetLong(&findProps, GS_FIND_MATCH_MODE, GS_MODE_CASS);
GsPropSetLong(&findProps, GS_FIND_STREET_OFFSET, 50);
GsPropSetLong(&findProps, GS_FIND_CENTERLINE_OFFSET, 0);
GsPropSetStr (&findProps, GS_FIND_CLIENT_CRS, "NAD83");
GsPropSetBool(&findProps, GS_FIND_ADDRCODE, TRUE);
GsPropSetBool(&findProps, GS_FIND_Z_CODE, FALSE);
GsPropSetBool(&findProps, GS_FIND_FINANCE_SEARCH, TRUE);
GsPropSetBool(&findProps, GS_FIND_MIXED_CASE, TRUE);
switch(GsFindWithProps(gs, &findProps)) {
case GS_SUCCESS:
GsDataGet(gs, GS_OUTPUT, GS_ADDRLINE, address, sizeof(address));
break;
case GS_ERROR:
case GS_ADDRESS_NOT_FOUND:
case GS_ADDRESS_NOT_RESOLVED:
case GS_LASTLINE_NOT_FOUND:
default:
fprintf(stderr, "Error geocoding address.\n");
}
GsFormatCASSHeader
Writes a CASS 3553 report to the header buffer argument.
Syntax
GsFunStat GsFormatCASSHeader(GsId gs,GsExtendCASSData *pData,int dataSize,pstr headerBuffer,int bufSize);
Arguments
GsIdgs ID returned by GsInitWithProps for the current instance of GeoStan. Input.
GsExtendCASSData*pData Writes the CASS information to the header buffer using the data passed in GsExtendCASSData. This structure contains the following (lengths are in brackets):
char CASS_Z4CompanyName[40] |
Name of the company requesting certification. Input. |
char CASS_Z4Config[4] |
CASS Z4Change configuration. Input. |
char CASS_Z4SoftwareName[30] |
Name of the CASS Z4Change software. Input. |
char DPV_FileFormat |
DPV date file format: F - Full, S - Split, and L - Flat. Input. |
char certificationDate[24] |
Date of certification. Input. |
intlu DPVDatabaseDate |
DPV database date. Input. |
intlu EWS_Denial |
Number of matches denied due to a matching address found in Ews.txt. Input. |
char listName[20] |
Name of the list. Input. |
char listProcessorName[25] |
Name of the list processor. Input. |
intlu LOTDatabaseDate |
eLOT database date. Input. |
char LOT_DPCCompanyName[40] |
Name of the company requesting eLOT & DPC Utility certification. Input. |
char LOT_DPCConfig[4] |
eLOT & DPC Utility configuration. Input. |
char LOT_DPCSoftwareName[30] |
Name of the eLOT & DPC Software. Input. |
char LOTVersion[12] |
eLOT software version. Input. |
char version[12] |
Software version. Input. |
intlu nCarrt |
Number of Carrier Routes coded records. Input. |
intlu nDpbc |
Number of delivery point coded records. Input. |
intlu nHighRiseDefault |
Number of records that matched to the default highrise record. Input. |
intlu nHighRiseExact |
Number of records that matched to an exact highrise record. Input. |
intlu nLACS |
Number of LACSLink converted addresses, if LACSLink is loaded. If LACSLink is not loaded, the number of records that match to a LACS ZIP + 4 record. Input. |
intlu nLot |
Number of eLOT coded records. Input. |
intlu nRecs |
Number of processed records. Input. |
intlu nRuralRouteDefault |
Number of records matched to a default rural route record. Input. |
intlu nRuralRouteExact |
Number of records matched to an exact rural record. Input. |
intlu nSuiteLink |
Number of records processed through SuiteLink. Input. |
intlu nTotalDPV |
Deprecated. Number of DPV confirmed addresses. Input. |
intlu nZIP |
Number of records with an output 5-digit ZIP Code. Input. |
intlu nZIP4 |
Number of records with an output ZIP+4. (number of DPV confirmed records). Input. |
intlu nZ4Changed |
Number of records skipped (not reprocessed) because the ZIP + 4 did not change. Input. |
char pSearchPath[256] |
Location of GeoStan data. Input. |
intlu structVersion |
Set equal to GS_GEOSTAN_VERSION. Input. |
char templateName[256] |
Full path and name of cass3553.frm template file. Input. |
intlu zip4DatabaseDate |
ZIP + 4 database date. Input. |
char z4ChangeVersion[12] |
Z4Change software version. Input. |
intdataSize Size of the CASS report data structure. Input.
pstrheaderBuffer Buffer containing the CASS header line from the Stage file. Input, Output.
intbufSize Length of the header buffer. Input.
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx for more information.
GS_WARNING Call GsErrorGetEx for more information.
Prerequisites
GsInitWithProps()
Notes
When you specify a version number for either version, z4ChangeVersion or LOTVersion, GeoStan updates the corresponding fields in the header buffer similar to GsWriteExtendCASSReport. For example, entering a version number for z4ChangeVersion prompts GeoStan to fill the following fields in cass3553.frm:
Section "B. List", Item "2b": Date List Processed Z4Change
Section "B. List", Item "3b": Date of Database Product Used Z4Change
Section "C. Output", Item "1b": Total Coded Z4Change Processed
To develop CASS certified applications in GeoStan, you must have the correct license agreement with Precisely. You must also obtain CASS certification from the USPS for your application. Using GeoStan does not make an application CASS certified. For information on becoming CASS certified, refer to Appendix E: CASS certification overview
This data is defined in geostan.h in the data structure GsExtendCASSData.
Example
/* assign outCASSHeader flag to process->writeCASSHeader */
/* open address input file */
/* if writeCASSHeader flag is set to 1 read and store headerline first */
/* otherwise ignore it */
if(process->writeCASSHeader == 1)
{
process->headerBuffer = (pstr)malloc(process->inRecLen + 1);
/* get the headerline */
fgets(process->headerBuffer, process->inRecLen,process->inputFile);
/* write the header line as is to the output file as a spaceholder */
fwrite(process->headerBuffer, process->inRecLen, 1, process->outputFile);
fputs("\n", process->outputFile);
}
.
.
.
/* Geocode each address line and write its output record to the output file */
.
.
.
/* if writeCASSHeader flag is set to 1 -> write the CASS header to the output file... */
if(process->writeCASSHeader == 1)
{
/* copy the data from CASSData structure into the correct positions in the header as defined by USPS */
GsFormatCASSHeader(process->gs, &CASSData, sizeof( CASSData ), process->headerBuffer, process->inRecLen);
/* set the file pointer to the beginning of the file */
fseek(process->outputFile, 0L, SEEK_SET);
/* - write the formatted header line to the output file */
fwrite(process->headerBuffer, process->inRecLen, 1,process->outputFile);
}
GsFormatDpvFalsePosDetail
Formats a DPV false-positive detail record from GsDpvGetFalsePosDetail.
Syntax
GsFunStat GsFormatDpvFalsePosDetail(GsId gs,GsDpvFalsePosDetailData *pData, int structSize,pstr detail,int detailLength);
Arguments
GsIdgs ID returned by GsInitWithProps for the current instance of GeoStan. Input.
GsDpvFalsePosDetailData *pData Pointer to the DPV report data structure. This value is completed by GsDpvGetFalsePosDetail().
intstructSize Size of the GsDpvFalsePosDetail data structure. Input.
pstrdetail Buffer containing the DPV false-positive detail record after GsFormatDpvFalsePosDetail() successfully completes. When the GeoStan application writes the false-positive report, it writes this buffer to the last line of the file. Output.
intdetailLength Length of the detail buffer. Input.
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx() for more information.
GS_WARNING Call GsErrorGetEx() for more information.
Prerequisites
GsDpvGetFalsePosDetail()
GsFormatDpvFalsePosHeader
Syntax
GsFunStat GsFormatDpvFalsePosHeader(GsId gs,GsFalsePosHeaderData *pData,int structSize,pstr header,int headerLength);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsFalsePosHeaderData *pData Pointer to the DPV report data structure. This value is completed by GsDpvGetFalsePosHeaderStats(). Input.
intstructSize Size of the GsFalsePosHeaderData data structure. Input.
pstrheader Buffer containing the DPV false-positive header after GsFormatDpvFalsePosHeader() successfully completes. When the GeoStan application writes the false-positive report, it writes this buffer to the first line of the file. Output.
intheaderLength Length of the header buffer. Input.
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx() for more information.
GS_WARNING Call GsErrorGetEx() for more information.
Prerequisites
GsInitWithProps() with appropriate DPV initialization properties set.
GsDpvGetFalsePosHeaderStat()
GsFormatLACSFalsePosDetail
Formats a LACSLink false-positive detail record from GsLACSGetFalsePosDetail.
Syntax
GsFunStat GsFormatLACSFalsePosDetail(GsId gs,GsFalsePosDetailData *pData,int structSize,pstr detail,int detailLength);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsFalsePosDetailData *pData Pointer to the LACSLink report data structure. This value is completed by GsLACSGetFalsePosDetail(). Input.
intstructSize Size of the LACSLink report data structure. Input.
pstrdetail Buffer containing the LACSLink false-positive detail record after GsFormatLACSFalsePosDetail() successfully completes. When the GeoStan application writes the false-positive report, it writes this buffer to the last line of the file. Output.
intdetailLength Length of the detail buffer. Input.
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx() for more information.
GS_WARNING Call GsErrorGetEx() for more information.
Prerequisites
GsLACSGetFalsePosDetail()
GsFormatLACSFalsePosHeader
Syntax
GsFunStat GsFormatLACSFalsePosHeader(GsId gs,GsFalsePosHeaderData *pData, int structSize,pstr header,int headerLength);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsFalsePosHeaderData *pData Pointer to the LACSLink report data structure. This value is completed by GsLACSGetFalsePosHeaderStats(). Input.
intstructSize Size of GsFalsePosHeaderData data structure. Input.
pstr header Buffer containing the LACSLink false-positive header after GsFormatLACSFalsePosHeader() successfully completes. When the GeoStan application writes the false-positive report, it writes this buffer to the first line of the file. Output.
intheaderLength Length of the header buffer. Input.
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx() for more information.
GS_WARNING Call GsErrorGetEx() for more information.
Prerequisites
GsLACSGetFalsePosHeaderStats()
GsGetCoordsEx
Retrieves coordinates for the street segment found via GsFind.
Syntax
ints GsGetCoordsEx(GsId gs, pintl pCoords, intsu maxPoints);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
pintlpCoords Array of coordinates, in x,y (longitude, latitude) order. Output.
intsumaxPoints Maximum number of points to return; used to prevent writing past the end of the pCoords buffer. Input.
Return Values
Number of points assigned to buffer.
Prerequisites
GsFindWithProps()
Notes
This function returns an array of coordinates for the current feature found via GsFind().
GeoStan can return a maximum of 64 coordinate pairs, each pair consisting of two long integers.
Pass in a value of 2 for the maxPoints parameter in order to return the end points of the segment matched.
GeoStan returns the coordinates to the full precision stored in the GSD file and scales coordinate pairs to integers. For example, GeoStan returns a point at (-98.3, 29.7) as (983000, 297000). This is a different scale from that expected by Spatial+ and similar GIS applications, which typically express coordinates in millionths of degrees. You may need to scale coordinates obtained with this function before using them as input to other software libraries or applications.
GsGetDBMetadata
Populates the supplied buffer with the information about the item being queried for the specified database.
Syntax
ints GsGetDBMetadata(GsId gs, int WhichItem, int WhichDB, pstr buffer, int bufSize);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
intWhichItem Specific information to return. The following table includes types of available information. Input.
Mode |
Data Returned |
---|---|
GS_STATUS_DATATYPE_NUM |
Returns a numeric constant representing the data type. |
GS_STATUS_DATATYPE_STR |
Returns GS_SUCCESS or GS_ERROR. GeoStan places this retrieved information in the buffer. |
GS_STATUS_DATUM_NUM |
Returns a numeric constant representing the datum. |
GS_STATUS_DATUM_STR |
This is the NAD used natively by the data. It does not reflect the datum currently in use by GeoStan. See GS_FIND_CLIENT_CRS for further information on setting the NAD for geocoding. Returns GS_SUCCESS or GS_ERROR. The retrieved information is placed in the buffer. |
GS_STATUS_DB_COPYRIGHT |
Returns copyright information for User Dictionary. |
GS_STATUS_DB_COUNTRY |
Returns country associated with User Dictionary. |
GS_STATUS_DB_FILE_PATH |
Returns filesystem path to the User Dictionary. |
GS_STATUS_DB_VERSION |
Returns version string for the User Dictionary. |
intWhichDB A database index, starting at 0 and less than the number of databases returned by GsGetNumDB(). Input.
pstrbuffer Location to store the returned data. Output.
intbufSize Maximum size of data for GeoStan to return. Geostan.h lists as constraints the recommended buffer sizes for each item. These sizes include the null terminator and are the maximum lengths required to get the full output string. You can allocate a buffer that is smaller or larger than these values. However, if bufSize is shorter than the returned data, GeoStan truncates the data and does not generate an error. Input.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsInitWithProps()
GsGetLibVersion
Returns the current version of the GeoStan library.
Syntax
GsFunStat GsGetLibVersion( );
Arguments
None.
Return Values
Low Byte Major Version number.
High Byte Minor Version number.
Prerequisites
GsInitWithProps()
Notes
In general, the major version number changes whenever Precisely adds a new API features, or when the data structures in the GeoStan data files change.
GsGetMbr
Returns an MBR for the specified geographic element.
Syntax
GsFunStat GsGetMbr(GsId gs, GsEnum which, void *pData,long *pMinLong, long *pMinLat, long *pMaxLong,long *pMaxLat);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsEnumwhich The following table contains the possible values to which GeoStan assumes pData is pointing.
GS_MBR_SEGMENT |
GsSegmentHandle. |
GS_MBR_STREET |
GsStreetHandle. |
GS_MBR_CITY |
pstr, containing digits in the format SSFFFFCCC where S is state, F is finance, and C is city. |
GS_MBR_FINANCE |
pstr, containing digits in the format SSFFFF where S is state, and F is finance |
voidpData Points at value passed for which. Input.
longpMinLong Minimum longitude. One of four values that make up the MBR. Output.
longpMinLat Minimum latitude. One of four values that make up the MBR. Output.
longpMaxLong Maximum longitude. One of four values that make up the MBR. Output.
longpMaxLat Maximum latitude. One of four values that make up the MBR. Output.
Return Values
GS_ERROR For GS_MBR_SEGMENT and GS_MBR_STREET, if the specified geographical element has no spatial data, then all outputs have a value of zero. For GS_MBR_CITY and GS_MBR_FINANCE, if GeoStan cannot find the specified geographical element all outputs have a value of 0 (zero).
GS_SUCCESS The four longs hold the requested MBR.
Prerequisites
GsFindFirstStreet() and GsCityDataGet()
Notes
GeoStan handles all geocodes in these functions in ten-thousandths of degrees—not millionths like GsDataGet().
To make a call with GS_MBR_CITY, you must first make a call to GsPrepareIndexFinance(). To retrieve an MBR for GS_MBR_CITY, you must have already installed from the data media, or built the uscity.gsx file using the batchind.exe utility program. Use GsDataGet() with GS_QCITY to get a city number or a finance number (finance number is the first 6 digits of the city number).
MBR Representation by Lat/Lon
See GsFindFirstSegmentByMbr for an MBR representation by latitude and longitude.
GsGetNumDB
Returns the number of loaded databases.
Syntax
intlu GsGetNumDB(GsId gs);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsInitWithProps()
GsHandleGet
Retrieves data for objects found via GsFindFirst() and GsFindNext().
Syntax
GsFunStat GsHandleGet(GsId gs, GsEnum fSwitch,GsRangeHandle *pRange, pstr pBuffer,intsu bufLen);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsEnumfSwitch Enum for the argument you wish to retrieve. Input.
GsRangeHandle*pRange Pointer to the current range handle. Input.
pstrpBuffer Location to store the returned data. Output.
intsubufLen Maximum size of the data that GeoStan returns. If bufLen is shorter than the data returned by GeoStan, GeoStan truncates the data and does not generate an error. Input.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsFindFirst() or GsFindNext()
Notes
This function retrieves data from the geocode buffer for a given range handle. If you have a street or segment handle, you must convert the handle to a range handle before you can use this function.
See Appendix B: Extracting Data from GSD Files for more information on the Find First and Find Next searches. For information on extracting Alias information, refer to the description in GsMultipleGet().
GsHandleGetCoordsEx
Retrieves the coordinates for a street segment object found via GsFindFirst() and GsFindNext().
Syntax
ints GsHandleGetCoordsEx(GsId gs, GsSegmentHandle *pHandle,pintl pCoords, intsu maxPoints);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsSegmentHandle*pHandle Handle of the segment object for the coordinates returned. Input.
pintlpCoords Array of coordinates, in x,y (longitude, latitude) order. Output.
intsumaxPoints Maximum number of points that GsGetCoordsEx() returns; used to prevent writing past the end of pCoords buffer. Input.
Return Values
Number of points assigned to the buffer.
Prerequisites
GsFindFirst() or GsFindNext()
Notes
This function returns an array of coordinates for the segment to which handle points.
GeoStan can return a maximum of 64 coordinate pairs, each pair consisting of two long integers.
Pass in a value of 2 for the maxPoints parameter in order to return the end points of the segment matched.
Example
/*This example retrieves coordinates for a street segment found using GsFindFirstSegment.*/
#define MAX_POINTS 50
.
.
.
ints NumCoords;
intl Coords[MAX_POINTS *2];
NumCoords = GsHandleGetCoordsEx(gs, &hSegment, Coords, MAX_POINTS);
for (int i = 0; i < NumCoords * 2; i += 2)printf("Lon = %ld, Lat = %ld\n", Coords[i], Coords[i+1]);
GsInitWithProps
Initializes GeoStan using properties.
Syntax
GsId GsInitWithProps(PropList* pInitProps, PropList* pStatusProps);
Arguments
PropList*pInitProps* Pointer to property list structure of type GS_INIT_PROP_LIST_TYPE. Input.
PropList*pStatusProps Pointer to property list structure of type GS_STATUS_PROP_LIST_TYPE. Output.
Return Values
Returns the ID of the GeoStan instance that was initialized.
Prerequisites
GsPropListCreate()andGsPropSet*()
Notes
Initializes GeoStan using the property list. The application owns the property lists. The status property list is guaranteed to contain properties for all defined status properties with their values properly set.
GsInitWithProps can be used to initialize GeoStan, DPV, and LACSLink with a single call (if the appropriate initialization properties are in the init list). When this function successfully completes, it populates the GS_INIT_GS_ID property in the property list referred to by the pInitProps parameter with the actual GeoStan ID.
If you invoke this function with the GeoStan ID property pre-set to a valid GeoStan ID, it will not attempt to re-initialize GeoStan. This is how GsInitWithProps() can be used to initialize DPV and/or LACSLink after GeoStan has already been initialized with a previous call to GsInitWithProps(). If you intend to reuse the same initialization property list to initialize GeoStan several times you must reset the GS_INIT_GS_ID property back to zero, or completely remove the property from the list. This informs GeoStan that you want a new GeoStan instance when you call GsInitWithProps().
These initialization properties are required for GsInitWithProps to function correctly:
GS_INIT_LICFILENAME
GS_INIT_DATAPATH
GS_INIT_PASSWORD
These initialization properties are recommended, but not required:
GS_INIT_Z4FILE (required for ZIP centroid matching)
GS_INIT_OPTIONS_Z9_CODE (required for ZIP centroid matching)
GS_INIT_CACHESIZE (to improve GeoStan performance)
GS_INIT_OPTIONS_ADDR_CODE (required for address matching)
GS_INIT_OPTIONS_SPATIAL_QUERY (required for reverse geocoding and the MBR-related functions)
All other available initialization properties are entirely optional. See tables GsInitWithProps properties.
Deprecation note:
On 32-bit systems, GsInitWithProps() will also populate the GS_INIT_GEOSTAN_ID with the GeoStan ID. However, the same is not true for 64-bit systems. As a result, the GS_INIT_GEOSTAN_ID property has been deprecated. Instead, use the GS_INIT_GS_ID property for getting/setting the GeoStan ID.
Example
GsFunStat retval;
PropList initProps;
PropList statusProps;
GsPropListCreate( &initProps, GS_INIT_PROP_LIST_TYPE);
GsPropListCreate( &statusProps, GS_STATUS_PROP_LIST_TYPE);
GsPropSetStr( &initProps, GS_INIT_LICFILENAME, "c:\\test\\Data\\my.lic");
GsPropSetStr(&initProps, GS_INIT_DATAPATH, "c:\\test\\Data" );
GsPropSetStr(&initProps, GS_INIT_Z4FILE, ""c:\\test\\Data\\us.z9" );
GsPropSetLong(&initProps, GS_INIT_PASSWORD, 12345678 );
GsPropSetLong(&initProps, GS_INIT_GSVERSION, GS_GEOSTAN_VERSION );
GsPropSetBool(&initProps, GS_INIT_OPTIONS_ADDR_CODE, TRUE );
GsPropSetBool(&initProps, GS_INIT_OPTIONS_Z9_CODE, TRUE );
GsPropSetBool(&initProps, GS_INIT_OPTIONS_SPATIAL_QUERY, TRUE );
GsPropSetLong(&initProps, GS_INIT_CACHESIZE, 0 );
GsPropSetLong(&initProps, GS_INIT_FILE_MEMORY_LIMIT, 4000 );
GsPropSetLong(&initProps, GS_INIT_GEOSTAN_ID, 0 ); // not required, but demonstrates the usage.
gs = GsInitWithProps(&initProps, &statusProps);
GsLACSClearStats
Clears the LACSLink statistics.
Syntax
GsFunStat GsLACSClearStats(GsId gs,GsLACSCompleteStats *pStats,int structSize);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsLACSCompleteStats*pdata This structure retrieves LACSLink statistics since the application initialized LACSLink. This structure contains the following:
intlu nTotalProcessed Total number of records processed through LACSLink. Output.
intlu nTotalAMatches Total number of records matched through LACSLink. Output.
intlu nTotal09Matches Total number of records converted through LACSLink, but no new address provided. Output.
intlu nTotal00Matches Total number or records not matched through LACSLink. Output.
intlu nTotal14Matches Total number of records converted through LACSLink. Output.
intlu nTotal92Matches Total number of records matched through LACSLink where GeoStan dropped the unit number. Output.
intstructSize Size of the GsLACSCompleteStats data structure. Input.
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx() for information.
Prerequisites
GsInitWithProps() with appropriate LACSLink initialization properties set.
GsLACSGetCompleteStats
Syntax
GsFunStat GsLACSGetCompleteStats(GsId gs,GsLACSCompleteStats *pdata,int structSize);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsLACSCompleteStats*pdata This structure retrieves LACSLink statistics since the application initialized LACSLink. This structure contains the following:
intlu nTotalProcessed Total number of records processed through LACSLink. Output.
intlu nTotalAMatches Total number of records matched through LACSLink. Output.
intlu nTotal09Matches Total number of records converted through LACSLink, but no new address provided. Output.
intlu nTotal00Matches Total number or records not matched through LACSLink. Output.
intlu nTotal14Matches Total number of records converted through LACSLink. Output.
intlu nTotal92Matches Total number of records matched through LACSLink where GeoStan dropped the unit number. Output.
intstructSize Size of the GsLACSCompleteStats data structure. Input.
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx() for information.
GS_WARNING Call GsErrorGetEx() for information.
Prerequisites
GsInitWithProps() with appropriate LACSLink initialization properties set.
GsLACSGetFalsePosDetail
Retrieves the detail record for a LACSLink false-positive report.
Syntax
GsFunStat GsLACSGetFalsePosDetail(GsId gs,GsFalsePosDetailData *pdata,int structSize);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsFalsePosDetailData*pdata This structure retrieves the LACSLink detail record for false-positive address match using the data passed in GsFalsePosDetailData. The data members are details provided by GeoStan for the false-positive report. This structure contains the following:
char AddressSecondaryAbbreviation Unit type (APT, SUITE, LOT).Output.
char AddressSecondaryNumber Unit number.Output.
char AddressPrimaryNumber House number.Output.
char Filler Reserved for future implementation. Output.
char MatchedPlus4 ZIP Code extension.Output.
char MatchedZIPCODE ZIP Code.Output.
char StreetName Name of the street.Output.
char StreetpreDirectional Street name predirectional (N, S, E, W).Output.
char StreetPostDirectional Street name postdirectional (N, S, E, W).Output.
char StreetSuffixAbbreviation Street type (AVE, ST, RD).Output.
intstructSize Size of the GsFalsePosDetailData data structure. Input.
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx() for more information.
GS_WARNING Call GsErrorGetEx() for more information.
Prerequisites
GsInitWithProps() with appropriate LACSLink initialization properties set. GS_LACSLINK_IND == "F"
GsLACSGetFalsePosHeaderStats
Syntax
GsFunStat GsLACSGetFalsePosHeaderStats(GsId gs,GsFalsePosHeaderData *pdata, int structSize);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsFalsePosHeaderData*pdata This structure retrieves the LACSLink header record for false-positive address matches using the data passed in GsFalsePosHeaderData. The output data members are statistics for the false-positive report. The input data members include information to correctly complete the false-positive report for the USPS. This structure contains the following:
intlu nTotalRecordsMatched Number of records matching through LACSLink. Output.
intlu nTotalRecordsProcessed Number of records processed through LACSLink. Output.
char MailersAddressLine Address of the mailer. Input.
char MailersCityName City of the mailer. Input.
char MailerCompanyName Name of the mailer. Input.
char MailersStateName State of the mailer. Input.
char Mailers9DigitZip 9-digit ZIP Code of the mailer. Input.
intstructSize Size of the GsFalsePosHeaderData data structure. Input.
Return Values
GS_SUCCESS
GS_ERROR Call GsErrorGetEx() for more information
GS_WARNING Call GsErrorGetEx() for more information.
Prerequisites
GsInitWithProps() with appropriate LACSLink initialization properties set. GS_LACSLINK_IND == "F"
GsMultipleGet
Returns the address elements for the match candidate specified.
Syntax
GsFunStat GsMultipleGet(GsId gs, GsEnum fSwitch, ints index,pstr pBuffer, intsu bufLen);
Arguments
GsIdgs ID returned by GsInitWithProps for the current instance of GeoStan. Input.
GsEnumfSwitch Enum for the argument you want to retrieve. Input.
intsindex Entry number (0-based) of the possible match. Input.
pstrpBuffer Location for the returned data. Output.
intsubufLen Maximum size of data for GeoStan to return. If bufLen is shorter than the data returned by GeoStan, GeoStan truncates the data and does not generate an error. Input.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsNumMultiple()
Notes
This function retrieves data from the GeoStan buffer for match candidates. GeoStan indicates a match candidate as the GS_ADDRESS_NOT_RESOLVED return code for GsFindWithProps.() It is important to first test for an intersection match, since the enums are different for retrieving intersection and non-intersection matches.
When using any street name enum (GS_NAME, GS_PREDIR, GS_POSTDIR, GS_TYPE), an additional modifier is available. You can use GS_ALIAS to request specific alias information, rather than preferred name information. For example in Boulder, CO, Wallstreet is an alias for Fourmile Canyon. 123 Wallstreet, Boulder CO 80301, matches to 123 Fourmile Canyon Dr.
GsMultipleGet( ..., GS_NAME,... ) returns "FOURMILE CANYON". GsMultipleGet( ...,GS_ALIAS | GS_NAME,... ) returns "WALLSTREET".
If you use GS_ALIAS with an enum that does not return alias information (such as GS_ZIP), GeoStan returns the information in the normal format. If GS_IS_ALIAS returns A07, you can only get information based on the returned address, not the alias.
When using GsDataGet(), returned results may be altered for the best match based on all the candidates found. GsMultipleGet() however does not change the results but provides raw data for the returned candidates. Therefore, some enums may differ from GsMultipleGet() and GsDataGet(). For example, GS_ADDRLINE, only contains information available from the candidate data records. If there is no unit low-high on the candidate, no unit information is added to the GS_ADDRLINE.
The following enums are different if the GsDataGet() spatial information is inferred against another candidate in the list:
GS_LAT |
GS_LON |
GS_LOC_CODE |
|
These enums differ when CASS logic decides the city/ ZIP/ ZIP+4 on the GsDataGet() lastline versus pure candidate information used for GsMultipleGet():
GS_CITY |
GS_CITY_SHORT |
GS_LASTLINE |
GS_MM_RESULT_CODE |
GS_MATCH_CODE |
GS_NAME_CITY |
GS_URB_NAME |
GS_ZIP |
GS_ZIP_CARRTSORT |
GS_ZIP_CITYDELV |
GS_ZIP_CLASS |
GS_ZIP_FACILITY |
GS_ZIP_UNIQUE |
GS_ZIP4 |
GS_ZIP9 |
GS_ZIP10 |
These enums differ when information from the input carries over on the GsDataGet() versus pure candidate information used for GsMultipleGet():
GS_ADDRLINE |
GS_FIRM_NAME |
GS_HOUSE_NUMBER |
GS_UNIT_NUMBER |
GS_UNIT_TYPE |
|
Example
/* This example prints information about possible matches. It assumes that GsFindWithProps returned GS_ADDRESS_NOT_RESOLVED. */
char buffer[60];
int bInter;
printf( "Possibles:\n" );
/* Test for match candidate and set bInter to non-zero if true. */
GsMultipleGet( gs, GS_INTERSECTION, 0, buffer,sizeof(buffer) );
bInter = *buffer == 'T';
/* Get the number of multiples and print them as we loop through each one. */
int n = GsNumMultiple( gs );
for ( int i = 0; i < n; ++i )
{printf( "%d: ", i );
/* If we don't have an intersection, print the ranges. */
if ( !bInter )
{
GsMultipleGet( gs, GS_LORANGE, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_HIRANGE, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
}
/* Print the street name for both intersections and non intersections.*/
GsMultipleGet( gs, GS_PREDIR, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_NAME, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_TYPE, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_POSTDIR, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
/* If an intersection match, print the second street name. */
if ( bInter )
{
printf( " AT " );
GsMultipleGet( gs, GS_PREDIR2, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_NAME2, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_TYPE2, i, buffer, sizeof(buffer) );
printf("%s", buffer );
GsMultipleGet( gs, GS_POSTDIR2, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
}
/* Print each possible on a new line. */
printf( "(%s)\n", buffer );
}
GsMultipleGetHandle
Returns the range handle for the match candidate specified.
Syntax
GsFunStat GsMultipleGetHandle(GsId gs,ints index,GsRangeHandle *pHandle);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
intsindex Entry number (0-based) of the possible match. Input.
GsRangeHandle *pHandle Range handle for the possible match entry. Output.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsNumMultiple()
Notes
This function can extract additional information about a possible match than GsMultipleGet(). It retrieves the range handle for the possible match indicated by the entry argument. Once you have the correct range handle, you can retrieve information by using GsHandleGet(). For a list of elements returned by GsMultipleGet() or GsHandleGet(), see Enums for storing and retrieving data.
GsNumMultiple
Returns the number of match candidates found.
Syntax
GsFunStat GsNumMultiple(GsId gs);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
Return Values
Positive value indicates the number of match candidates. GS_ERROR indicates an error.
Prerequisites
GsFind()
Notes
This function returns the number of match candidates found. Use GsMultipleGet() to retrieve the actual address elements for each possible match. A return code from GsFindWithProps() indicates a possible match.
Example
See GsMultipleGet.
GsPrepareIndexFinance
Prepares GSX files needed to cover the finance area.
Syntax
GsFunStat GsPrepareIndexFinance(GsId gs,long finance,const char *outDir,void *progressData,spatialQueryProgress progress);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
longfinance Finance area to index. In the format ssffff, where s=state and f=finance. Input.
const charoutDir Directory to write .gsx files. Input.
void*progressData Data to pass to progress. Input.
spatialQueryProgressprogress Callback function for progress meter. If NULL GeoStan does not call progress. Input.
Return Values
GS_SUCCESS
GS_ERROR
GS_CANCEL Progress function had a cancel request from the user.
GS_NOT_FOUND More than 6 digits were entered for the finance parameter.
Prerequisites
GsCityDataGet()
Notes
Prepares the reverse geocoding system for searching the requested finance area, unlike PrepareIndexMbr(), which prepares the system for each finance area included partially or wholly within the area bounded by the four input points. See PrepareIndexMbr for more information.
Call before GsFindFirstSegmentByMbr().
This function expects that you have either installed the prebuilt GSX file that ships on the data media or that you have already created the GSX file using the batchind.exe utility included with GeoStan. For more information, refer to the GeoStan Geocoding Suite Utilities Reference Manual.
For proper execution, initialize GeoStan with a call to GsInitWithProps() and include at least the following properties set to TRUE:
GS_INIT_OPTIONS_ADDR_CODE
GS_INIT_OPTIONS_SPATIAL_QUERY
GS_INIT_OPTIONS_Z9_CODE
In addition, when using GsInitWithProps() include the outDir directory in the search path. For example, C:\data;C:\gsxout, where C:\data contains the primary data files and C:\gsxout is the output directory for GSX files. Include finmbr.dat in the GsInitWithProps() search path when creating GSX files.
See GsPrepareIndexMbr for more information on the progress function.
GsPrepareIndexMbr
Prepares GSX files needed to cover the MBR.
Syntax
GsFunStat GsPrepareIndexMbr(GsId gs,long lon1,long lat1,long lon2,long lat2,const char *outDir,void *progressData,spatialQueryProgress progress);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
longlon1 Minimum longitude. One of four values that make up the MBR. Input.
longlat1 Minimum latitude. One of four values that make up the MBR. Input.
longlon2 Maximum longitude. One of four values that make up the MBR. Input.
longlat2 Maximum latitude. One of four values that make up the MBR. Input.
const char *outDir Directory to write .gsx files.
void *progressData Data to pass to progress. Input.
spatialQueryProgressprogress Callback function for progress meter. If NULL GeoStan does not call progress. Input.
Return Values
GS_SUCCESS
GS_ERROR
GS_CANCEL Progress function received a cancel request from user.
Prerequisites
GsInitWithProps()
MBR Representation by Lat/Lon
See GsFindFirstSegmentByMbr for an MBR representation by latitude and longitude.
Notes
Prepares the reverse geocoding system for each Finance Area needed to cover the MBR specified by the four lat/lon parameters.
Call before GsFindFirstSegmentByMbr().
For proper execution, initialize GeoStan with a call to GsInitWithProps and include at least the following properties set to TRUE:
GS_INIT_OPTIONS_ADDR_CODE
GS_INIT_OPTIONS_SPATIAL_QUERY
GS_INIT_OPTIONS_Z9_CODE
In addition, when using GsInitWithProps() include the outDir directory in the search path. For example, C:\data;C:\gsxout, where C:\data contains the primary data files and C:\gsxout is the output directory for .gsx files. Include finmbr.dat in the GsInitWithProps() search path when creating .gsx files.
Example
See GsFindFirstSegmentByMbr for an example.
Progress Function
You can use the progress function as a progress counter and to cancel spatial queries interactively. The progress function is defined in geostan.h as follows:
intl (*spatialQueryProgress)(void * param, intl mode, intl value);
void *param Points at the progressData argument passed to the GsPrepareIndexMbr() function.
intlmode One of three values in the following table. Value contents vary depending on mode.
0 |
Initialize progress meter.value is estimated total number of steps. |
Return GS_PROGRESS_CONTINUE or GS_PROGRESS_CANCEL. |
1 |
value contains current step number. |
Return GS_PROGRESS_CONTINUE or GS_PROGRESS_CANCEL. |
2 |
Terminate progress meter.value is unused. |
Return value ignored. |
GsPropFind
Finds a property in a property list.
Syntax
GsFunStat GsPropFind(PropList* pProps, PropEnum pPropID);
Arguments
PropList*pProps Pointer to property list structure. Input.
PropEnumpPropID The ID of the property to find. Input.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsPropListCreate()
Notes
GS_SUCCESS returns if the property is found in the property list. GS_ERROR returns if the property is not found.
Example
GsFunStat retval;
PropList findProps;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropSetBool(&findProps, GS_FIND_MIXED_CASE, TRUE);
retval = GsPropFind(&findProps, GS_FIND_Z9_CODE);
retval = GsPropFind(&findProps, GS_FIND_MIXED_CASE);
GsPropFirst
Sets property iterator to first property.
Syntax
GsFunStat GsPropFirst(Proplist* pProps);
Arguments
Proplist*pProps Pointer to property list structure. Input.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsPropListCreate()
Notes
Prior to iterating through the properties in a property list, this function sets the property list iterator to the beginning of the list.
Example
GsFunStat retval;
PropList findProps;
PropEnum propID;
PropValue propValue = {GS_UNDEF_PROP_TYPE, 0};
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropSetBool(&findProps, GS_FIND_MIXED_CASE, TRUE);
retval = GsPropSetLong(&findProps, GS_FIND_MATCH_MODE, GS_MODE_CLOSE);
retval = GsPropSetLong(&findProps, GS_FIND_STREET_OFFSET, 50);
GsPropFirst(&findProps );
while (GsPropGetNext(&findProps, &propID, &propValue) == GS_SUCCESS ) {
switch (propValue.propType) {
case GS_BOOL_PROP_TYPE:
...
break;
case GS_LONG_PROP_TYPE:
...
break;
case GS_STRING_PROP_TYPE:
...
break;
case GS_DOUBLE_PROP_TYPE:
case GS_UNDEF_PROP_TYPE:
default:
...
break;
}
}
GsPropGetBool
Retrieves a boolean property.
Syntax
GsFunStat GsPropGetBool (PropList* pProps, PropEnum propID,qbool *pbValue);
Arguments
PropList*pProps Pointer to property list structure. Input.
PropEnum propID The property ID. Input.
qbool*pbValue The boolean value of the property. Output.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsPropListCreate()
GsPropGetDouble
Retrieves a double property.
Syntax
GsFunStat GsPropGetDouble (PropList* pProps, PropEnum propID,double *pdValue);
Arguments
PropList*pProps Pointer to property list structure. Input.
PropEnumpropID The property ID. Input.
double*pbValue The double value of the property. Output.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsPropListCreate()
GsPropGetInfo
Retrieves information about a property.
Syntax
GsFunStat GsPropGetInfo(PropEnum propID, int* pPropType, PropListType* pPropListType, pstr pPropName, int* pPropCategory);
Arguments
PropEnumpropID The property ID. Input.
int*pPropType Output.
PropListType*pPropListType Output.
pstrpPropName Output.
int*pPropCategory Output.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsPropListCreate()
GsPropGetLong
Retrieves an integer property.
Syntax
GsFunStat GsPropGetLong(Proplist* pProps, PropEnum propID,intl *plValue);
Arguments
Proplist*pProps Pointer to property list structure. Input.
PropEnumpropID The property ID. Input.
intl*plValue Long value. Output.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsPropListCreate()
Example
GsFunStat retval;
PropList findProps;
long lValue;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropSetLong(&findProps, GS_FIND_MATCH_MODE, GS_MODE_CLOSE);
retval = GsPropGetLong(&statusProps, GS_FIND_MATCH_MODE, &lValue);
GsPropGetNext
Iterates to the next sequential property in the property list.
Syntax
GsFunStat GsPropGetNext(Proplist* pProps, PropEnum* propID,PropValue pPropValue);
Arguments
Proplist*pProps Pointer to property list structure. Input.
PropEnum*propID The property ID. Output.
PropValuePropValue Pointer to union of property values. Output.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Notes
GeoStan only populates one of the PropValue union members. The populated union member depends on the property output type. If there is no "next" entry, GeoStan returns an error.
Example
See GsPropFirst example.
GsPropGetPtr
Retrieves a pointer property.
Syntax
GsFunStat GsPropGetPtr(PropList* pProps, PropEnum propID,void *pValue);
Arguments
PropList*pProps Pointer to property list structure. Input.
PropEnumpropID The property ID. Input.
void*pValue The pointer value. Output.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsPropListCreate()
GsPropGetStr
Retrieves a string property.
Syntax
GsFunStat GsPropGetStr(Proplist* pProps, PropEnum propID,pstr pBuffer, intsu bufLen);
Arguments
Proplist*pProps Pointer to property list structure. Input.
PropEnumpropID The property ID. Input.
pstrpBuffer String buffer. Output.
intsubufLen Length of a string buffer. Input.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Example
GsFunStat retval;
PropList findProps;
char buffer[256];
const int bufLen = 256;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropSetStr(&findProps, GS_FIND_CLIENT_CRS, "NAD83");
retval = GsPropGetStr(&findProps, GS_FIND_CLIENT_CRS, buffer, bufLen);
GsPropGetStrLen
Retrieves the length of the string value of a property.
Syntax
GsFunStat GsPropGetStrLen (Proplist* pProps, PropEnum propID,intlu bufLen);
Arguments
Proplist*pProps Pointer to property list structure. Input.
PropEnumpropID Property ID. Input.
intlubufLen Required string buffer length. Output.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites()
GsPropListCreate()
GsPropListClear
Clears (empties) a property list.
Syntax
GsFunStat GsPropListClear(PropList* pProps);
Arguments
PropList*pProps Pointer to property list structure. Input and Output.
Return Values
GS_ERROR
GS_SUCCESS
Example
GsFunStat retval;
PropList findProps;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropSetBool(&findProps, GS_FIND_Z_CODE, TRUE);
retval = GsPropSetLong(&findProps, GS_FIND_STREET_OFFSET, 50);
retval = GsPropListClear(&findProps);
Notes
You may want to clear a property list for re-populating from the beginning with new properties.
GsPropListCreate
Creates and initializes a property list to contain GeoStan initialization, status, or find properties.
Syntax
GsFunStat GsPropListCreate (Proplist* pProps, PropListType type);
Arguments
Proplist*pProps Pointer to property list structure. Input and Output.
PropListTypetype Type of property list. Input.
Return Values
GS_ERROR
GS_SUCCESS
Notes
This function creates/initializes a property list for use in GsInitWithProps() or GsFindWithProps(). Any property list created by this function needs to eventually be destroyed with GsPropListDestroy().
Example
GsFunStat retval;
PropList initProps;
PropList statusProps;
PropList findProps1;
PropList findProps2;
/* For initialization of GeoStan */
GsPropListCreate(&initProps, GS_INIT_PROP_LIST_TYPE);
GsPropListCreate(&statusProps, GS_STATUS_PROP_LIST_TYPE);
/* For standard property default preference */
retval = GsPropListCreate(&findProps1, GS_FIND_PROP_LIST_TYPE);
/* For custom property default preference */
retval = GsPropListCreate(&findProps2, GS_FIND_PROP_LIST_TYPE);
GsPropListDestroy
Destroys a property list.
Syntax
GsFunStat GsPropListDestroy (Proplist* pProps);
Arguments
Proplist*pProps Pointer to property list structure. Input and Output.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Notes
Find property lists cannot be destroyed until all searching is complete. Any list created by GsPropListCreate() must eventually be destroyed with GsPropListDestroy().
Example
GsFunStat retval;
PropList findProps;
retval = GsPropListCreate( &findProps, GS_FIND_PROP_LIST_TYPE );
retval = GsPropListDestroy ( &findProps );
GsPropListGetCount
Retrieves the number of properties in a property list.
Syntax
GsFunStat GsPropListGetCount (Proplist* pProps, intl* pCount);
Arguments
Proplist*pProps Pointer to property list structure. Input.
intl*pCount Returned count. Output.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Example
intl count;
GsFunStat retval;
PropList findProps;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropListGetCount(&findProps, &count);
GsPropListGetType
Retrieves the type of the property list.
Syntax
GsFunStat GsPropListGetType(Proplist* pProps, PropListType* pType);
Arguments
Proplist*pProps Pointer to property list structure. Input.
PropListType*pType The property list type. Output.The following table contains valid enums.
GS_UNDEF_PROP_LIST_TYPE |
Undefined |
GS_INIT_PROP_LIST_TYPE |
Initialization property list |
GS_FIND_PROP_LIST_TYPE |
Find property list |
GS_STATUS_PROP_LIST_TYPE |
Status property list |
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Example
GsFunStat retval;
PropList findProps;
PropListType type;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropListGetType(&findProps, &type);
GsPropListRead
Reads the property list from a file or character string.
Syntax
GsFunStat GsPropListRead(Proplist* pProps, gs_const_str infile, gs_const_str pBuffer);
Arguments
Proplist*pProps Pointer to property list structure. Output.
gs_const_str infile File containing the list properties, or NULL. Input.
s_const_strpBuffer String buffer containing the properties to read if infile is NULL. Input.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Notes
If the infile argument value is "stdin", then this function reads the properties from standard in. If the infile argument is NULL, then this function reads the properties from the pBuffer string.
Example
GsFunStat retval;
PropList findProps;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropListRead(&findProps, "testprop.txt", NULL);
GsPropListReset
Resets the property list to its default state.
Syntax
GsFunStat GsPropListReset(PropList* pProps);
Arguments
PropList*pProps Pointer to property list structure. Input and Output.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Notes
Depending on the type of property list, this might mean "empty" (init and status properties) or refill it with some needed defaults (find properties).
Example
GsFunStat retval;
PropList findProps;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropSetBool(&findProps, GS_FIND_Z_CODE, TRUE);
retval = GsPropSetLong(&findProps, GS_FIND_STREET_OFFSET, 50);
retval = GsPropListReset(&findProps);
GsPropListWrite
Writes the property list to a file or a character string.
Syntax
GsFunStat GsPropListWrite(PropList* pProps, gs_cont_str outfile,pstr pBuffer, intsu bufLen);
Arguments
PropList*pProps Pointer to property list structure. Input.
gs_cont_str outfile File containing the list properties, or NULL. Output.
pstrpBuffer String buffer to write to outfile is NULL. Output.
intsubufLen Length of pBuffer (writing should not exceed bufLen). Input.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Notes
If the outfile argument value is "stdout", then this function writes the properties to standard out. If the outfiles argument is NULL, then this function writes the properties to the pBuffer string.
Example
GsFunStat retval;
PropList findProps;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropSetBool(&findProps, GS_FIND_Z_CODE, TRUE);
retval = GsPropSetLong(&findProps, GS_FIND_STREET_OFFSET, 50);
retval = GsPropListWrite(&writeProps, "testprop.txt", NULL, 0);
GsPropRemove
Removes a property from the property list.
Syntax
GsFunStat GsPropRemove(PropList* pProps, PropEnum propID);
Arguments
PropList* pProps Pointer to property list structure. Input and Output.
PropEnumpropID Property ID. Input.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Example
GsFunStat retval;
PropList findProps;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropSetBool(&findProps, GS_FIND_Z_CODE, TRUE);
retval = GsPropRemove(&findProps, GS_FIND_Z_CODE);
GsPropSetAsStr
Sets a property where input name and value are both strings.
Syntax
GsFunStat GsPropSetAsStr(PropList* pProps, gs_const_str pszPropName, PropEnum propID, gs_const_str pszValue);
Arguments
PropList*pProps Pointer to property list structure. Input and Output.
gs_const_str pszPropName The string name of the property to set. Input.
PropEnumpropID Property ID. Input.
gs_const_strpszValue The string value of the property. Input.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Notes
This function performs the conversion to correct property enum ID and property value type. If the input property name is a NULL pointer, the property ID is for use in identifying the property instead. If both property name and property ID are present, preference is given to the property name, ignoring the property ID.
Example
GsFunStat retval;
PropList initProps;
retval = GsPropListCreate(&initProps, GS_INIT_PROP_LIST_TYPE);
retval = GsPropSetAsStr(&initProps, "GS_INIT_PASSWORD", GS_UNDEFINED_PROPID, "12345678");
retval = GsPropSetAsStr(&initProps, NULL, GS_INIT_PASSWORD, "12345678");
GsPropSetBool
Sets a boolean property.
Syntax
GsFunStat GsPropSetBool(PropList* pProps, PropEnum propID,qbool value);
Arguments
PropList*pProps Pointer to property list structure. Input and Output.
PropEnumpropID Property ID. Input.
qboolvalue Boolean value. Input.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Example
GsFunStat retval;
PropList findProps;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropSetBool(&findProps, GS_FIND_MIXED_CASE, TRUE);
GsPropSetDouble
Sets a double property.
Syntax
GsFunStat GsPropSetDouble (PropList* pProps, PropEnum propID,double value);
Arguments
PropList*pProps Pointer to property list structure. Input and Output.
PropEnumpropID Property ID. Input.
doublevalue Double value. Input.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
GsPropSetLong
Sets an integer property.
Syntax
GsFunStat GsPropSetLong (PropList* pProps, PropEnum propID, intl value);
Arguments
PropList* pProps Pointer to property list structure. Input and Output.
PropEnumpropID Property ID. Input.
intlValue Long value. Input and Output.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Example
GsFunStat retval;
PropList findProps;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropSetLong(&findProps, GS_FIND_MATCH_MODE, GS_MODE_CASS);
GsPropSetPtr
Sets a pointer property.
Syntax
GsFunStat GsPropSetPtr(PropList* pProps, PropEnum propID, void *pValue);
Arguments
PropList* pProps Pointer to property list structure. Input and Output.
PropEnumpropID Property ID. Input.
void*pValue Pointer value. Input.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate
GsPropSetStr
Sets a string property.
Syntax
GsFunStat GsPropSetStr (PropList* pProps, PropEnum propID, gs_const_str value);
Arguments
PropList*pProps Pointer to property list structure. Input and Output.
PropEnumpropID Property ID. Input.
gs_const_strvalue String value. Input.
Return Values
GS_ERROR
GS_SUCCESS
Prerequisites
GsPropListCreate()
Example
GsFunStat retval;
PropList findProps;
retval = GsPropListCreate(&findProps, GS_FIND_PROP_LIST_TYPE);
retval = GsPropSetStr(&findProps, GS_FIND_CLIENT_CRS, "NAD83");
GsSetSelection
Allows you to select a match from a set of match candidates.
Syntax
GsFunStat GsSetSelection(GsId gs, ints index);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
intsindex Index number (0-based) of the possible match. Input.
Return Values
GS_SUCCESS
GS_ERROR Selection is out of range.
Prerequisites
GsMultipleGet()
Notes
After GsFindWithProps() returns GS_ADDRESS_NOT_RESOLVED, use GsNumMulitple() and GsMultipleGet() to determine which possible match is the correct match. Then use GsSetSelection() to load that possible match into the data retrieval buffers and retrieve it using GsDataGet().
Example
/* This example prints information about possible matches. It assumes that GsFind returned GS_ADDRESS_NOT_RESOLVED. */
char buffer[60];
int bInter;
printf( "Possibles:\n" );
/* Test for match candidate and set bInter to non-zero if true. */
GsMultipleGet( gs, GS_INTERSECTION, 0, buffer,sizeof(buffer) );
bInter = *buffer == 'T';
/* Get the number of multiples and print them as we loop through each one. */
int n = GsNumMultiple( gs );
for ( int i = 0; i < n; ++i )
{
printf( "%d: ", i );
/* If we don't have an intersection, print the ranges. */
if ( !bInter )
{
GsMultipleGet( gs, GS_LORANGE, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_HIRANGE, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
}
/* Print the street name for both intersections and nonintersections. */
GsMultipleGet( gs, GS_PREDIR, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_NAME, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_TYPE, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_POSTDIR, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
/* If an intersection match, print the second street name. */
if ( bInter )
{
printf( " AT " );
GsMultipleGet( gs, GS_PREDIR2, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_NAME2, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
GsMultipleGet( gs, GS_TYPE2, i, buffer, sizeof(buffer) );
printf("%s", buffer );
GsMultipleGet( gs, GS_POSTDIR2, i, buffer, sizeof(buffer) );
printf( "%s ", buffer );
}
/* Print each possible on a new line. */
printf( "(%s)\n", buffer );
}
int index = 0;
GsFunStat funStat;
printf(" Please enter selection number.");
scanf("%i", &index);
funStat = GsSetSelection(gs, index);
if (funStat == GS_SUCCESS)
{
GsDataGet( gs, GS_OUTPUT, GS_FIRM_NAME, firm, sizeof(firm) );
GsDataGet( gs, GS_OUTPUT, GS_ADDRLINE, address, sizeof(address) );
GsDataGet( gs, GS_OUTPUT, GS_LASTLINE, lastline, sizeof(lastline) );
GsDataGet( gs, GS_OUTPUT, GS_LON, longitude, sizeof(longitude) );
GsDataGet( gs, GS_OUTPUT, GS_LAT, latitude, sizeof(latitude) );
printf( "%s\n"
"%s\n"
"%s\n"
"longitude: %lf\n"
"latitude: %lf\n", firm, address, lastline, /* ascii data */
(double)(atof(longitude)/1000000.0), /* normalize longitude */
(double)(atof(latitude)/1000000.0) ); /* normalize latitude */
}
else
printf("Address information not found.");
GsSetSelectionRange
Allows GeoStan to use a record found outside of GsFind as a match.
Syntax
GsFunStat GsSetSelectionRange(GsId gs, GsRangeHandle *pRange,GsEnum options);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsRangeHandle*pRange Range pointer of the object to return as a match. A range record indicates a range of addresses such as "2800 - 2900 Center Green Drive". Input.
GsEnumoptions GsSetSelectionRange options. The following table contains the valid enums. Input.
GS_ADDR_CODE |
Attempts to standardize and find an address geocode. You must set this switch for address standardization. If this switch is not set, GeoStan uses only the input ZIP and ZIP + 4 address elements. |
GS_WIDE_SEARCH |
GeoStan considers all records matching the first letter of the street name, rather than the soundex key on the street name. This results in a wider search. |
GS_FINANCE_SEARCH |
When you add GS_FINANCE_SEARCH, GeoStan searches the entire Finance Area for possible streets. These modifiers have no meaning when doing a ZIP centroid match. |
GS_Z9_CODE |
Attempts to find ZIP + 4 centroid match only (no ZIP+2 or ZIP). |
GS_Z7_CODE |
Attempts to find ZIP+2 centroid match only (no ZIP + 4 or ZIP). |
GS_Z5_CODE |
Attempts to find a ZIP centroid match (no ZIP + 4 or ZIP+2). |
GS_Z_CODE |
Attempts to find a match based on all possible ZIP centroids available. |
If you specify GS_ADDR_CODE and a ZIP centroid option, GeoStan only returns a ZIP Code centroid match if an address geocode is not available.
You must use either GS_ADDR_CODE or GS_Z_CODE or both.(In general, you should specify both.) These option settings are additive.
If you enter a pre-parsed address, it must contain the USPS abbreviations for street type, predirectionals, and postdirectionals.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsFindFirstRange()
Notes
This function allows you to get a range handle that points to the address range to which you want to match. Use this command to indicate that GsFindWithProps() found the match, when in fact the match is set to whichever range handle is passed through the *pRange argument. You must specify the options enum so that subsequent calls to GsDataGet() have the type of location information to return.
This function returns standardized results from a list generated by GsFindFirst/GsFindNext. Use this function to perform a query that lets the user choose from a list of possible matches.
GsSoundex
Generates a soundex key for use in GsFindFirst.
Syntax
intlu GsSoundex(pstr pName);
Arguments
pstrpName String to convert to a soundex key. Input.
Return Values
Soundex key.
Notes
This function generates a soundex key for a street name. Use it in conjunction with GsFindFirstStreet() when you want to perform a soundex search. The soundex key, when combined with a locale (state, and city), is the primary index into the GeoStan databases. Searching by soundex allows you to use your own scoring and matching mechanism for geocoding.
GeoStan uses a modified version of the standard soundex algorithm first published by Donald Knuth. The modifications employed within GeoStan include special treatment of certain prefixes such as MAC, KN, and WR; numeric street names; and an encoding scheme to pack the key into the smallest number of bits.
GsTerm
Terminates GeoStan.
Syntax
GsFunStat GsTerm(GsId gs);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
Return Values
GS_SUCCESS
GS_ERROR
Notes
You must call GsTerm() at the conclusion of a session to close files and release other resources. It closes GeoStan and invalidates GsId.
You cannot call any function that accepts a GsId parameter except GsInit_r following a call to GsTerm. Also, you should only delete the initialization, find, and status properties (by calling GsPropListDestroy) that you have used with GeoStan after the call to GsTerm.
GsTestRange
Determines whether a house number falls within a range.
Syntax
GsFunStat GsTestRange(GsId gs, gs_const_str number,gs_const_str rangeLo, gs_const_str rangeHi);
Arguments
GsIdgs ID returned by GsInitWithProps for the current instance of GeoStan. Input.
gs_const_strnumber House number to test. Input.
gs_const_strrangeLo Low house number in the range. Input.
gs_const_strrangeHi High house number in the range. Input.
Return Values
Non-zero Number is within the range.
0 Number is not within the range or GeoStan cannot make the comparison.
Prerequisites
GsInitWithProps()
Notes
GsTestRange() tests to see if the house number is within the range defined by rangeHi and rangeLo. It handles all valid house number patterns, such as
|
|
|
|
|
|
|
|
|
|
:
This function uses USPS rules defined in Publication 28, "Postal Addressing Standards," to determine if a number is in range. For more information, refer to this publication on the USPS Website.
Some patterns are not comparable with other patterns; for example, 123 is not comparable to a range of 1A01 to 1A99.
GsVerifyAuxiliaryRecord
Validates an auxiliary file record.
Syntax
GsFunStat GsVerifyAuxiliaryRecord (GsId gs, const char *pRecord)
Arguments
GsIdgs ID returned by GsInitWithProps for the current instance of GeoStan. Input.
const char*pRecord Pointer to a string buffer containing one auxiliary file record. Input.
Return Values
GS_SUCCESS The record is valid and GeoStan will load the record. This includes comment records; records whose first character is a semicolon.
GS_WARNING The record contains a non-fatal error and GeoStan will load the record. Because this record has a problem, an input address may not be able to match to the record in its current state, or the output data from a match to the record may not be valid.
GS_ERROR The record contains a fatal error and GeoStan will NOT load the file.
Prerequisites
GsInitWithProps()
Example
char buffer[800];
char msg1[GS_MAX_STR_LEN], msg2[GS_MAX_STR_LEN];
FILE * fp;
GsId gs;
GsFunStat rc;
int recno = 0;
/* open the GAX file */
fp = fopen( GAXfilename, "r" );
if ( fp )
{
/* get the first record from the file */
fgets( buffer, sizeof(buffer), fp );
/* while not EOF, process each record */
while( !feof(fp) )
{
recno++;
/* verify the record */
rc = GsVerifyAuxiliaryRecord( gs, buffer );
if ( rc != GS_SUCCESS )
{
/* the record is not perfect, so get more information */
while ( GsErrorHas(gs) )
{
GsErrorGetEx( gs, msg1, msg2 );
printf( "%s %d: %s %s\n", rc == GS_ERROR ? "FATAL ERROR" : "WARNING", recno, msg1, msg2 );
}
printf("\n");
}
/* get the next record from the file */
fgets( buffer, sizeof(buffer), fp );
}
}
GsVerifyGSDDataPresent
Validates the presence of data for specified states in the GSD data file.
Syntax
GsFunStat GsVerifyGSDDataPresent (GsId gs, GsStateList states)
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsStateListstates Structure containing state abbreviations and/or FIPS codes. Input.
Return Values
GS_SUCCESS All states or FIPS codes specified in states are present in GSD data.
GS_GSD_DATA_MISSING One or more states or FIPS codes specified in states are not present in GSD data.
GS_ERROR states structure is invalid.
Prerequisites
You must create a valid GsId with a call to GsInitWithProps().
If GeoStan did not find the GSD primary data for all the specified states, this function creates an error in the error list retrievable using GSErrorGetEx. The error message code is 114 and the error message is "NO GSD DATA FOUND FOR STATE" and indicates which states GeoStan did not find.
The state abbreviations and corresponding state FIPS codes are as follows:
State |
Abbrev. |
FIPSCodea |
State |
Abbrev. |
FIPSCodea |
---|---|---|---|---|---|
Alabama |
AL |
1 |
New Jersey |
NJ |
34 |
Alaska |
AK |
2 |
New Mexico |
NM |
35 |
Arizona |
AZ |
4 |
New York |
NY |
36 |
Arkansas |
AR |
5 |
North Carolina |
NC |
37 |
California |
CA |
6 |
North Dakota |
ND |
38 |
Colorado |
CO |
8 |
Ohio |
OH |
39 |
Connecticut |
CT |
9 |
Oklahoma |
OK |
40 |
Delaware |
DE |
10 |
Oregon |
OR |
41 |
District of Columbia |
DC |
11 |
Pennsylvania |
PA |
42 |
Florida |
FL |
12 |
Rhode Island |
RI |
44 |
Georgia |
GA |
13 |
South Carolina |
SC |
45 |
Hawaii |
HI |
15 |
South Dakota |
SD |
46 |
Idaho |
ID |
16 |
Tennessee |
TN |
47 |
Illinois |
IL |
17 |
Texas |
TX |
48 |
Indiana |
IN |
18 |
Utah |
UT |
49 |
Iowa |
IA |
19 |
Vermont |
VT |
50 |
Kansas |
KS |
20 |
Virginia |
VA |
51 |
Kentucky |
KY |
21 |
Washington |
WA |
53 |
Louisiana |
LA |
22 |
West Virginia |
WV |
54 |
Maine |
ME |
23 |
Wisconsin |
WI |
55 |
Maryland |
MD |
24 |
Wyoming |
WY |
56 |
Massachusetts |
MA |
25 |
American Samoa |
AS |
60 |
Michigan |
MI |
26 |
Guam |
GU |
66 |
Minnesota |
MN |
27 |
North Mariana Islands |
MP |
69 |
Mississippi |
MS |
28 |
Palau |
PW |
70 |
Missouri |
MO |
29 |
Puerto Rico |
PR |
72 |
Montana |
MT |
30 |
Virgin Islands |
VI |
78 |
Nebraska |
NE |
31 |
|
|
|
Nevada |
NV |
32 |
|
|
|
New Hampshire |
NH |
33 |
|
|
|
a: Do not specify Minor Islands (UM, 74) or you will receive an error. This is defined as a FIPS code, but the USPS does not generate data for this code. |
The following pseudo FIPS codes support APO and FPO addresses:
Address |
Abbrev. |
FIPS Code |
---|---|---|
Armed Forces Europe |
AE |
57 |
Armed Forces Pacific |
AP |
58 |
Armed Forces Americas |
AA |
59 |
GsVerifyGSDDataPresent() uses the GsStateList structure to pass in a list of state abbreviations or state FIPS codes to verify their presence in the GSD data. This structure contains the following:
typedef struct GsStateList
{
char *state[100]; /* array of 2 char null terminated strings */
int cnt; /* entries in 'state' that are filled in */
} GsStateList;
Example
typedef struct GsStateList
{
char state[100][3]; /* array of 2 char null terminated strings */
int cnt; /* entries in 'state' that are filled in */
}
GsStateList;
GsStateList states;
char error_buffer[256];
char details_buffer[256];
/* populate states structure with all states that are required to be in the GSD data in order to create a CASS report */
/* note that test for CASS required GSD data is not a fatal error so the 'error' flag is not set */
states.cnt = 0;
strcpy(states.state[states.cnt++], "AL");
strcpy(states.state[states.cnt++], "AK");
strcpy(states.state[states.cnt++], "AZ");
/* additional states can be copied into the array here */
....
strcpy(states.state[states.cnt++], "AA");
if (GsVerifyGSDDataPresent(process->gs, states) == GS_SUCCESS)
process->hasCASSRequiredData = 1;
else
{
process->hasCASSRequiredData = 0;
while ( GsErrorHas(process->gs))
GsErrorGetEx (process->gs, error_buffer, details_buffer);
printf ( "%s: %s\n", error_buffer, details_buffer ); /* report the errors */
}
GsWriteExtendCASSReport
Writes a USPS CASS report based on a specified template.
Syntax
GsFunStat GsWriteExtendCASSReport (GsId gs, GsExtendCASSData *pData, int dataSize,gs_const_str outputName);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
GsExtendCASSData*pData The structure allows you to specify the name of the template form. This structure is also defined in geostan.h. This function writes a CASS Report using the data passed in GsExtendCASSData. Input.
This structure contains the following:
char CASS_Z4CompanyName[40] Name of the company requesting certification.
char CASS_Z4Config[3] CASS Z4Change Configuration.
char CASS_Z4SoftwareName[30] Name of the CASS Z4Change software.
char DPV_FileFormat DPV date file format: F - Full, S - Split, and L - Flat. Input.
char certificationDate[24] Date of certification.
intlu DPVDatabaseDate DPV database date.
intlu EWS_Denial Number of matches denied due to a matching address found in Ews.txt.
char listName[20] Name of the list.
char listProcessorName[25] Name of the list processor.
intlu LOTDatabaseDate eLOT database date.
char LOT_DPCCompanyName[40] Name of the company requesting eLOT & DPC utility certification.
char LOT_DPCConfig[3] eLOT & DPC Utility Configuration.
char LOT_DPCSoftwareName[30] Name of the eLOT & DPC Software.
char LOTVersion[12] eLOT software version.
char version[12] Software version. Input.
intlu nCarrt Number of records that are Carrier Routes coded.
intlu nDpbc Number of records that are delivery point coded.
intlu nHighRiseDefault Number of records matched to a default highrise record.
intlu nHighRiseExact Number of records that matched to an exact highrise record. Input.
intlu nLACS Number of old (usually rural-route) addresses converted.
intlu nLot Number of records that are eLOT coded.
intlu nRecs Number of records processed.
intlu nRuralRouteDefault Number of records matched to a default rural route record.
intlu nRuralRouteExact Number of records matched to an exact rural record. Input.
intlu nSuiteLink Number of records processed through SuiteLink. Input.
intlu nTotalDPV Deprecated.
intlu nZIP4 Number of records with an output ZIP+4.
intlu nZIP Number of records with an output 5-digit ZIP Code.
intlu nZ4Changed Number of records skipped (not reprocessed) because the Z4 did not change.
char pSearchPath[256] Location of the GeoStan data.
intlu structVersion Set equal to GS_GEOSTAN_VERSION.
char templateName[256] Full path and name of the cass3553.frm template.
char version[12] CASS software version.
ntlu zip4DatabaseDate ZIP + 4 database date.
char z4ChangeVersion[12] Z4Change software version.
dataSize Size of the CASS report data structure. Input
outputName Path and name of the report file to write. Input.
Return Values
GS_SUCCESS
GS_ERROR
Prerequisites
GsInitWithProps()
Notes
Before running a CASS report, verify GeoStan has loaded the GSU, USPS.gdi, and GSZ files (ZIPMove data).
When you specify a version number for either version, Z4ChangeVersion or LOTVersion, GeoStan updates the corresponding fields in the template cass3553.frm file. For example, entering a version number for Z4ChangeVersion prompts GeoStan to fill these fields on cass3553.frm:
B. List, 2b. Date List Processed Z4Change
B. List, 3b. Date of Database Product Used Z4Change
C. Output, 1b. Total Coded Z4Change Processed
To develop CASS-certified applications in GeoStan, you must have the correct license agreement with Precisely. You must also obtain CASS certification from the USPS for every application. Using GeoStan does not make an application CASS certified.
To receive a return value of GS_SUCCESS, the only members of the GsExtendCASSData struct that you MUST set are structVersion and templateName.
Example
/* get the file status and file Date of required files */
fileRet = GsFileStatus(process->gs, fileStateCode, &fileDate);
/* if the file status is GS_GSL_EXISTS fill in the data structure */
if( fileRet & GS_GSL_EXISTS )
{
/* fill CASSData structure */
CASSData.structVersion = GsGetLibVersion();
CASSData.nRecs = report.records;
strcpy( CASSData.listName, outListName );
strcpy( CASSData.pSearchPath, process->searchPath );
strcpy( CASSData.LOTVersion, CASSData.version );
strcpy( CASSData.CASS_Z4CompanyName, "Precisely" );
strcpy( CASSData.LOT_DPCCompanyName, "Precisely" );
strcpy( CASSData.CASS_Z4Config, "CAS" );
strcpy( CASSData.LOT_DPCConfig, "CAS" );
strcpy( CASSData.CASS_Z4SoftwareName, programName );
/* if doing z4Change - assign z4ChangeVersion */
if (process->lastZ4ChangeProcessDate[0])
{
strcpy( CASSData.z4ChangeVersion, CASSData.version );
}
strcpy( CASSData.LOT_DPCSoftwareName, programName );
strcpy( CASSData.listProcessorName, "" );
CASSData.zip4DatabaseDate = fileDate;
CASSData.LOTDatabaseDate = fileDate;
}
.
.
.
/* calculate nRecs, nZip, nZip4... and set these in the CASSData structure */
Geocode( )
.
.
.
/* write the CASS report from data in CASSData structure */
retval = GsWriteExtendCASSReport( process->gs, &CASSData, sizeof( CASSData ), process->CASSFile );
if( retval != GS_SUCCESS )
{
printf( " Error Writing CASS Report! \n" );
}
GsZ4Changed
Determines if the USPS changed a ZIP + 4 address definition since GeoStan last processed the record.
Syntax
GsFunStat GsZ4Changed (GsId gs, pstr pZip, pstr pZip4, pstr pDate);
Arguments
GsIdgs ID returned by GsInitWithProps() for the current instance of GeoStan. Input.
pstrpZip First five digits of the 9-digit ZIP Code to test. Input.
pstrpZip4 Last four digits of the 9-digit ZIP Code to test. Input.
pstrpDate Date of the GeoStan ZIP + 4 information file used to process the record, in the format MMYYYY (for example, 081998). Input.
Return Values
GS_ERROR Usually occurs when GeoStan could not load the GSL file, because:
n GeoStan could not find the GSL file in the path
n The GSL file was a different release level than the GSD file.
GS_Z4_CHANGE
GS_Z4_NO_CHANGE
Prerequisites
GsInitWithProps()
Notes
For ZIP4Change to work, the GeoStan GSL file must be in a directory listed in the GS_INIT_DATAPATH initialization property of the property list that you pass when calling GsInitWithProps. The Z4Change file, which is generated by the USPS, contains a record for every ZIP + 4 in the country. Each record contains twelve flags that represent the last twelve months, starting with the current release date. Each of these flags has a value of either True or False, indicating if the ZIP + 4 changed for that monthly postal release. The GeoStan GSL file incorporates this information, which GsZ4Changed references.
Z4Change information is valuable to users who process very large address lists frequently. As you process each record, you can call GsZ4Changed and quickly tell if the record needs reprocessing. This information can help you quickly identify only those records that need reprocessing.
Your application must store the date of the GeoStan GSL file used to process a record. GeoStan uses this date as the GsZ4Changed pDate input parameter. This is the same date printed on the GeoStan DVD used for record processing, and is one month later that the release date of the USPS files used on the GeoStan DVD.
Use the following code example if you are unsure of the release date. You should run this code when standardizing a batch of records and store the resulting date string as input to GsZ4Changed in future processing runs.
Example
intsu postalDate;
int month, year;
char z4ChangeDate[7];
/* Get the postal release of the current files. */
GsFileStatus ( gs,"", &postalDate );
month = ((postalDate % 384) / 32) + 1;
year = (postalDate/384) + 1990;
/* Add one month to this date so it matches what is printed on the CD. */
if ( month !=12 )
{
++month;
}
else
{
++year;
month = 1;
}
/* Print the month and year to the date string in the format MMYYYY. */
sprintf( z4changedate, "%02d%d", month, year );