Example Node.js: Setting the Request Headers After Logging In
Your browser uses cookies as a means of authentication. However, the same cookies can also be set as HTTP Headers for authentication. The following Code Snippet shows how to use cookies to set your headers.
This code is written in JavaScript and runs directly from the command line
using Node.js. The request-promise
npm module is used as
for asynchronous handling.
There are three API calls being made in this example:
- Log in using credentials in the POST body.
-
GET a listing of repositories using the
ewtoken
from the previous call as a Request Header. -
Perform a search on a repository, using also the
epimresttoken
from the previous call.
<userName>
/
<password>
combination as shown in Lines 5 and
6 in the example below. These values were excluded from the example for
security purposes.You must install the request-promise
node module using the
command:
npm install request-promise
rp = require('request-promise')
reqHeader = {} // used as a request header
serverName = 'http://ewapp-stable.home'
userName = '--------'
password = '--------'
page = 1
pageSize = 200
rp.post( { uri: serverName+'/enable-api/login',
json : true,
body: {
login: userName,
password: password
}
})
.then( (authResp)=>{
reqHeader.ewtoken = authResp.token.token
return rp.get( { uri: serverName + '/webcm/rest/api/repositories?detail=true',
json: true,
headers: reqHeader } )
}).then( (repos)=>{
reqHeader.epimresttoken = repos.epimresttoken
let searchBody = {
"repositoryId": 10116,
"page": page,
"pageSize": pageSize
}
return rp.post({ uri: serverName + '/webcm/rest/api/items/search?forUI=false',
json: true,
headers: reqHeader,
body: searchBody })
.then((searchResult)=>{
console.log(`searchResult page=${page} length=${searchResult.data.itemList.length} of ${searchResult.data.searchTotal}`)
})
})
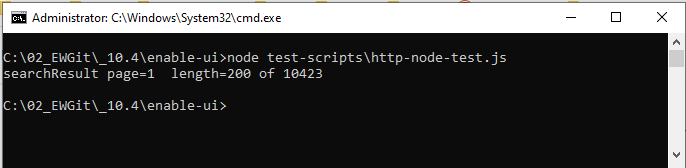
The epimresttoken
is the same token used to access the Swagger
pages for the API. Behind the scenes of the microservices
framework, this token is being managed on an as needed basis. If you have
a valid ewtoken
, then an epimresttoken
will be automatically created and set to your cookie.